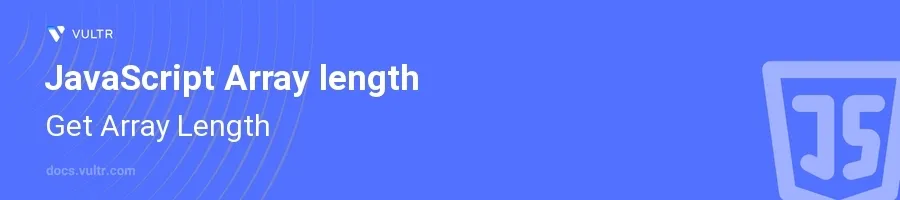
Introduction
In JavaScript, managing arrays efficiently is fundamental to developing interactive web applications. One of the most basic yet crucial properties when working with arrays is the length
property. It enables you to determine the number of elements in an array, which is essential for looping through arrays, manipulating their contents, and validating data.
In this article, you will learn how to effectively utilize the length
property in different scenarios. Explore how this property can be utilized to manipulate and interact with array data, ranging from simple operations like counting elements to more complex tasks like truncating arrays.
Retrieving the Length of an Array
Determining the Number of Elements
Declare an array with multiple elements.
Access the
length
property to retrieve the number of elements.javascriptconst fruits = ['Apple', 'Banana', 'Cherry']; console.log(fruits.length);
This code sample prints the length of the
fruits
array, which is3
, indicating there are three elements in the array.
Checking for Empty Arrays
Initially, define an empty array.
Use the
length
property to check if the array is empty.javascriptconst emptyArray = []; console.log(emptyArray.length === 0); // Outputs: true
Here, the expression
emptyArray.length === 0
evaluates totrue
, confirming that the array is indeed empty.
Modifying Array Length
Truncating an Array
Start with an array containing several elements.
Modify the
length
property to truncate the array.javascriptconst numbers = [1, 2, 3, 4, 5]; numbers.length = 3; console.log(numbers); // Outputs: [1, 2, 3]
Set
numbers.length = 3
. The elements beyond the third position are removed, truncating the array to contain only[1, 2, 3]
.
Dynamically Increasing Array Size
Begin with an array.
Increase the
length
property beyond the current number of elements.javascriptconst colors = ['red', 'blue']; colors.length = 4; console.log(colors); // Outputs: ['red', 'blue', undefined, undefined]
By setting
colors.length = 4
, the array now has two additionalundefined
slots, increasing the total length to four.
Conclusion
The length
property of JavaScript arrays is a powerful attribute that you can leverage to manage array sizes and manipulate their content. With it, you hold the key to performing a variety of operations—from simple tasks like counting elements and checking if an array is empty, to more advanced manipulations such as truncating arrays or artificially increasing their size. By mastering the length
property, enhance the functionality and performance of your JavaScript code in handling array data.
No comments yet.