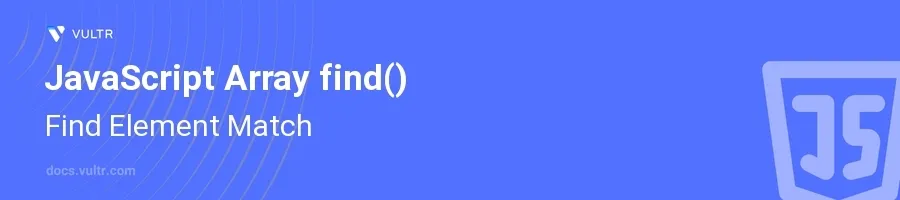
Introduction
The find()
method in JavaScript is an essential tool for searching through arrays to find the first element that meets a specific condition. This capability makes it invaluable in scenarios where you need to retrieve an item that matches particular criteria, from simple searches like finding a number in an array to more complex queries such as locating an object with specific properties within an array of objects.
In this article, you will learn how to effectively use the find()
method in various scenarios involving arrays. Discover how to implement it for different data types and how to manage cases where no matches are found.
Utilizing find() with Primitive Types
Find a Specific Number in an Array
Construct an array of numbers.
Use the
find()
method to locate a specific number.javascriptconst numbers = [5, 12, 8, 130, 44]; const found = numbers.find(element => element > 10); console.log(found);
This snippet finds the first number in the array
numbers
that is greater than 10. Since12
meets this condition, it is returned by thefind()
method.
Find a String by Condition
Create an array of strings.
Utilize the
find()
method to find a string that satisfies a certain condition.javascriptconst fruits = ['apple', 'banana', 'mango', 'orange']; const result = fruits.find(fruit => fruit.startsWith('m')); console.log(result);
In this code,
find()
searches for the first string that starts with the letter "m". The method returns 'mango' as it is the first string satisfying the condition.
Working with Objects in an Array
Locate an Object by Property
Define an array of objects where each object represents a person with properties like name and age.
Use
find()
to locate a person of a specific age.javascriptconst people = [ { name: 'John', age: 24 }, { name: 'Jane', age: 32 }, { name: 'Sam', age: 40 } ]; const person = people.find(individual => individual.age > 30); console.log(person);
Here, the
find()
method is used to locate the first person whose age is greater than 30. It returns the object{ name: 'Jane', age: 32 }
, which meets the specified condition.
Handling No Matching Elements
Consider what happens when there are no elements matching the condition.
Use the
find()
with a scenario highly likely to returnundefined
.javascriptconst smallNumbers = [1, 3, 5, 7, 9]; const nonExistent = smallNumbers.find(number => number > 10); console.log(nonExistent); // This will log 'undefined'
In this example, since no numbers are greater than 10,
find()
returnsundefined
. Recognize the importance of checks or fallbacks when dealing with possible non-matches.
Conclusion
The find()
function in JavaScript is a powerful method for locating elements in an array that satisfy a specific condition. It is particularly useful when working with data that involves searching through large arrays or extracting items based on unique attributes. By mastering the find()
method, you enhance your ability to handle data efficiently and effectively in your JavaScript projects, ensuring rapid access to relevant data. Through the examples and techniques discussed, leverage this function to keep your data processing concise and precise.
No comments yet.