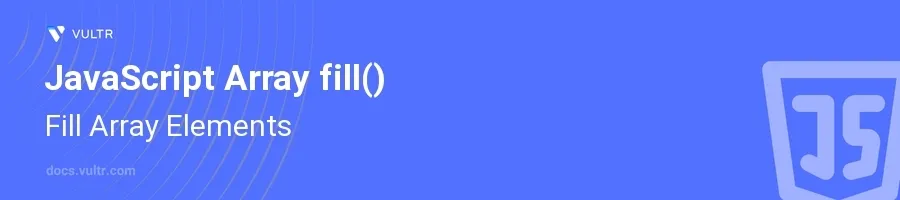
Introduction
The fill()
method in JavaScript provides an efficient way to fill all or a portion of an array with a static value. This method can be extremely useful when you need to initialize or reset array elements to a specific value without the overhead of manually setting each index in a loop. It applies directly to the original array, modifying its content inline and returning the modified array.
In this article, you will learn how to effectively utilize the fill()
method in various use cases, including filling an entire array, a portion of it, or using the method to quickly initialize newly created arrays.
Using fill() with Whole Arrays
Fill an Entire Array with a Static Value
Create an array.
Use the
fill()
method to fill it entirely with a predefined value, such as a number, string, or evennull
.javascriptlet myArray = new Array(5); myArray.fill("apple"); console.log(myArray);
This code initializes an array of five elements and fills each element with the string
"apple"
. When printed, the output will show all elements as"apple"
.
Utilize fill() for Array Initialization
Directly create and fill an array using chaining.
javascriptlet numArray = Array(3).fill(0); console.log(numArray);
Here, a new array with three elements is created and each element is initialized to
0
. This is a succinct way to initialize array elements to a specific value upon creation.
Using fill() with Array Subsections
Partially Fill an Array
Specify the start and end indices to appropriately use the
fill()
method within subsections of an array.javascriptlet partArray = [1, 2, 3, 4, 5]; partArray.fill("banana", 1, 3); console.log(partArray);
The
fill()
method in this example will replace elements from index 1 up to, but not including, index 3 with the string"banana"
. The output will demonstrate that only specific portions of the array are altered, showing[1, "banana", "banana", 4, 5]
.
Understand Boundary Effects
Learn how the
fill()
method handles boundary cases, such as when the end index exceeds the actual array length.javascriptlet boundaryArray = [10, 20, 30, 40, 50]; boundaryArray.fill("orange", 2, 10); console.log(boundaryArray);
In this snippet,
fill()
will replace elements starting at index 2 through the end of the array, despite the end index being out of bounds. The output array will be[10, 20, "orange", "orange", "orange"]
.
Conclusion
The fill()
method in JavaScript is a versatile tool for modifying arrays by setting all or parts of an array to a given value efficiently. It is particularly useful for initializing and resetting array data. By incorporating fill()
into your projects, you streamline operations related to array management, ensuring arrays are quickly adaptable to changes in data requirements. Utilize fill()
in various contexts, from setting up new data structures to dynamically updating existing ones, to enhance the effectiveness of your code.
No comments yet.