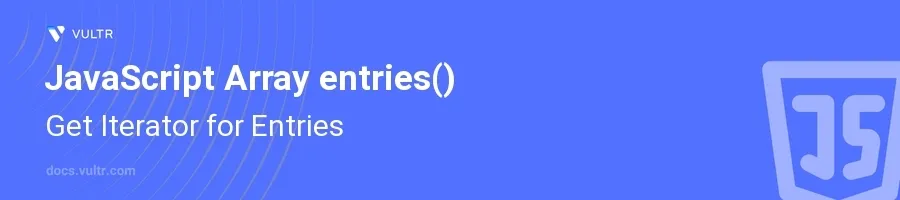
Introduction
The entries()
method in JavaScript is an essential tool when working with arrays, especially when you need access to both the index and the value of each array element. This method returns a new Array Iterator object that contains the key/value pairs for each index in the array, allowing for efficient and straightforward iteration.
In this article, you will learn how to effectively use the entries()
method in various scenarios. Explore how to iterate over arrays using this method, and understand how to integrate it with other JavaScript operations to facilitate complex data manipulations.
Using the entries() Method
Basic Usage of entries()
Define an array with several elements.
Call the
entries()
method to get the iterator.javascriptconst fruits = ["apple", "banana", "cherry"]; const iterator = fruits.entries();
This code initializes an iterator for the array
fruits
, where each item will be accessible as a pair of its index and value.
Iterating Over the Iterator
Use a
for...of
loop to iterate through the iterator returned byentries()
.javascriptfor (let [index, fruit] of fruits.entries()) { console.log(`Index ${index}: ${fruit}`); }
The
for...of
loop easily iterates through the index-value pairs, logging each fruit along with its index.
Integration with Destructuring
Combine the
entries()
method with array destructuring to handle elements in a more readable manner.javascriptconst foods = ["pizza", "burger", "pasta"]; let entriesIterator = foods.entries(); for (let [index, food] of entriesIterator) { console.log(`Food at index ${index}: ${food}`); }
Destructuring within the loop provides direct access to the index and value, enhancing the clarity and maintainability of the code.
Advanced Use Cases
Working with Spread Operator
Spread the iterator into an array to view all entries at once.
javascriptconst numbers = [1, 2, 3]; const allEntries = [...numbers.entries()]; console.log(allEntries);
By spreading the iterator, you can transform it back into an array of tuples, making it easier to visualize or manipulate all the entries in bulk.
Combining with Other Array Methods
Utilize
entries()
in conjunction with other array methods likemap()
orfilter()
for more complex transformations.javascriptconst names = ["Alice", "Bob", "Charlie"]; const filteredEntries = [...names.entries()].filter(([index, name]) => name.startsWith('A')); console.log(filteredEntries);
This example demonstrates filtering an array to find elements that start with the letter 'A', using the entries to preserve the original indices in the output.
Conclusion
The entries()
function in JavaScript is a versatile tool that facilitates not just simple iteration but also complex data manipulations, enhancing both the flexibility and readability of the code. By mastering the entries()
method along with its combination with loops and other JavaScript array methods, you can significantly improve the functionality and efficiency of your script. Utilize this method in various situations, from iterating through simple arrays to managing complex data structures, to maintain a clean and efficient codebase.
No comments yet.