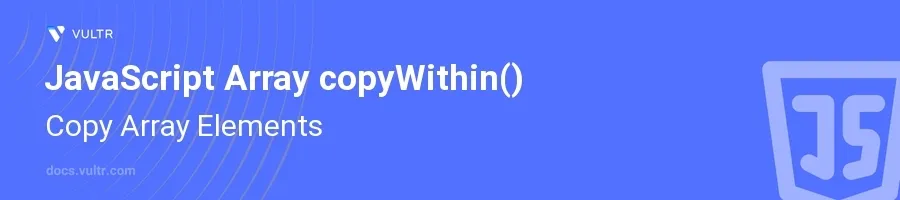
Introduction
The copyWithin()
method in JavaScript allows you to copy array elements within the same array to another location. This method is highly efficient for in-place rearrangement of elements and can be particularly useful for certain data manipulation tasks where you need to move or duplicate array segments without creating a new array.
In this article, you will learn how to use the copyWithin()
method in JavaScript in various scenarios. Explore how to use this method to manipulate and rearrange array data efficiently, understand its syntax, and see it in action through practical examples.
Understanding JavaScript’s copyWithin() Method
The Syntax of copyWithin()
copyWithin()
has a simple syntax:
array.copyWithin(target, start, end)
- The
target
is the index position to copy the elements to. - The
start
is the index position to start copying elements from. If omitted, it defaults to 0. - The
end
is the index up to which the elements should be copied (but not including the element at this index). If omitted, it defaults to the length of the array.
Basic Example of copyWithin()
Consider an array of integers.
Use
copyWithin()
to copy a part of the array to a different location within the same array.javascriptlet numbers = [1, 2, 3, 4, 5]; numbers.copyWithin(0, 3); console.log(numbers);
In this example, elements from index 3 to the end of the array are copied to start from index 0. The resulting array is
[4, 5, 3, 4, 5]
.
Practical Use Cases of copyWithin() in JavaScript
Reorganizing an Array
Sometimes, you might want to move parts of an array to the front or to another specific position.
Use copyWithin to shift elements efficiently.
javascriptlet fruits = ['apple', 'banana', 'cherry', 'date', 'fig']; fruits.copyWithin(1, 2); console.log(fruits);
In this example, elements starting from index 2 are copied starting from index 1. As a result, the elements
'cherry'
,'date'
, and'fig'
move forward, while'fig'
is duplicated.
Handling Large Arrays
In scenarios involving larger arrays,
copyWithin()
can be especially useful to avoid the overhead of removing and adding elements manually.Copy multiple elements within a large array.
javascriptlet largeArray = new Array(100).fill(0).map((_, index) => index + 1); largeArray.copyWithin(10, 30, 40); console.log(largeArray.slice(0, 50)); // Partial output for brevity
This example copies elements from indices 30 to 40 into positions starting at index 10. It works efficiently even for large arrays, making it a great option for performance-critical tasks.
Using copyWithin() to Reset Array Segments
copyWithin()
is also practical for duplicating or resetting segments of an array to the same value or sequence of values.Duplicate specific array segments.
javascriptlet resetArray = [1, 2, 3, 1, 2, 3]; resetArray.copyWithin(3, 0, 3); console.log(resetArray);
This duplicates the first half of the array over the second half, resulting in
[1, 2, 3, 1, 2, 3]
.
Conclusion
The copyWithin()
method in JavaScript is a powerful tool for in-place manipulation of arrays. It allows you to efficiently copy elements within an array without creating a new array. Whether you're reorganizing elements, handling large datasets, or resetting sections of an array, copyWithin()
provides a performance-optimized solution for these tasks. Mastering this method will significantly improve your ability to manage array data in JavaScript. Start incorporating copyWithin()
in your coding practices to see immediate improvements in your array handling tasks.
No comments yet.