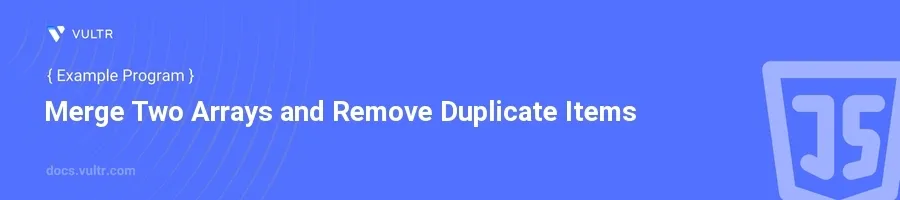
Introduction
Merging arrays in JavaScript and removing duplicates in the process is a common task encountered in many web development projects. This operation is useful when dealing with data that comes from multiple sources or when ensuring that a dataset contains only unique values.
In this article, you will learn how to merge two arrays in JavaScript and remove any duplicate items that may exist. The strategies explored will involve modern JavaScript methods that ensure a clean, efficient, and readable approach to solving this challenge. Dive into practical coding examples to grasp these techniques thoroughly.
Merging Arrays Using Set
Understanding Set
Recognize that a
Set
in JavaScript is a collection of unique values. By its very nature, it refuses to add a duplicate value.Utilize the
Set
object to handle unique operations automatically when merging two arrays.javascriptconst array1 = [1, 2, 3]; const array2 = [2, 3, 4, 5]; const mergedArray = [...new Set([...array1 , ...array2])]; console.log(mergedArray);
This snippet demonstrates merging
array1
andarray2
while removing duplicate values. The spread operator (...
) combines the arrays, andnew Set
ensures all elements are unique.
Set and Spread Operator Explanation
Understand that spreading both arrays inside a new
Set
combines each value once.Using another spread operator when assigning
mergedArray
converts theSet
back into an array.javascriptconst finalArray = [...mergedArray]; console.log(finalArray);
This code ensures that
mergedArray
, which is initially aSet
, is converted back to an array to work with usual array methods in JavaScript.
Merging Arrays Using Filter
Combining Arrays First
Conceive first merging the arrays into a single array without any filtration.
Utilize the
concat()
method or the spread operator to achieve this merge.javascriptconst array1 = [1, 2, 3]; const array2 = [2, 3, 4, 5]; const combinedArray = array1.concat(array2); console.log(combinedArray);
In this example, the
concat()
method mergesarray1
andarray2
intocombinedArray
, which might contain duplicates.
Removing Duplicates Using Filter
Apply the
filter()
method to remove duplicates fromcombinedArray
.Create a condition within
filter()
to only include items that have their first occurrence at the current index.javascriptconst uniqueArray = combinedArray.filter((item, index) => combinedArray.indexOf(item) === index); console.log(uniqueArray);
This code snippet filters out duplicates by checking if the current item's index is the first occurrence of that item in
combinedArray
. If it is, the item remains inuniqueArray
.
Practical Tips for Using These Methods
- Understand that using
Set
is generally more straightforward and often more efficient for merging arrays and removing duplicates. - Consider that
filter()
provides more flexibility if the merging criterion is more complex than just uniqueness. - Remember that using modern JavaScript techniques such as
Set
and array destructuring can drastically reduce the amount of code needed and enhance readability.
Conclusion
Merging two arrays and ensuring all items are unique in JavaScript can be efficiently accomplished using either the Set
object combined with the spread operator or the filter()
method with a uniqueness check. Both methods have their specific contexts where they shine in terms of readability, performance, and elegance. Harness these techniques to handle arrays cleanly and effectively in any JavaScript project, ensuring data integrity and optimization.
No comments yet.