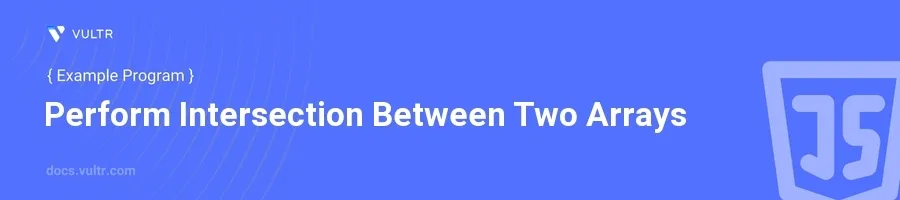
Introduction
In JavaScript, array operations such as intersection are commonly required tasks, especially in scenarios dealing with data manipulation or when you need to derive a set of common elements from multiple arrays. An intersection of two arrays results in a new array that contains only the elements that appear in both arrays, eliminating duplicates.
In this article, you will learn how to perform intersection operations between two arrays using JavaScript. Discover how to implement this functionality with clear examples, ensuring you can apply these techniques to various scenarios in your own projects.
Basic Intersection Using Filter and Includes
Define the Arrays and Perform Intersection
Define two arrays with some overlapping values.
Use the
filter()
method to iterate over one of the first array and theincludes()
method to check for common elements in the second array.javascriptconst array1 = [1, 2, 3, 4, 5]; const array2 = [4, 5, 6, 7, 8]; const intersection = array1.filter(item => array2.includes(item)); console.log(intersection);
This code snippet creates two arrays and filters
array1
to include only the elements that are also present inarray2
, resulting in an intersection of[4, 5]
. Thefilter()
method iterates through each item ofarray1
, and theincludes()
method checks if that item exists inarray2
.
Intersection Using Sets for Performance
Using Set to Handle Large Arrays
Convert both arrays to sets to utilize the fast lookup capabilities of sets.
Use the
Set
constructor for converting arrays and the spread operator for creating a new filtered array based on these sets.javascriptconst array3 = [1, 2, 2, 3, 4, 5, 5]; const array4 = [3, 4, 4, 5, 6, 7, 8]; const set1 = new Set(array3); const set2 = new Set(array4); const intersectionSet = [...set1].filter(item => set2.has(item)); console.log(intersectionSet);
Here, both
array3
andarray4
are converted into sets namedset1
andset2
respectively. Thefilter()
method is then applied to an array created fromset1
(using the spread operator), checking for the presence of each element inset2
using thehas()
method. The result is[3, 4, 5]
, an intersection of unique values from both arrays. This method is particularly effective for larger datasets sinceSet
operations are generally faster than array operations.
Advanced Intersection Techniques
Incorporating Multiple Arrays
Extend the intersection to include more than two arrays using a dynamic approach.
Use
reduce()
to generalize the intersection process for an array of arrays.javascriptconst arrays = [[1, 2, 3], [2, 3, 4], [3, 4, 5]]; const commonIntersection = arrays.reduce((acc, arr) => acc.filter(item => arr.includes(item))); console.log(commonIntersection);
In this example, the
arrays
variable houses several arrays. Thereduce()
method initiates the intersection process by taking the first array as the initial accumulator (acc
), then continuously filters it down by checking against each subsequent array usingfilter()
andincludes()
. This results in[3]
, showcasing the elements common to all provided arrays.
Conclusion
Performing array intersections in JavaScript is a vital skill in many development tasks, particularly useful in data processing or when working with complex datasets. By understanding and utilizing the methods demonstrated — from basic filtering with includes()
to more advanced techniques involving Set
and reduce()
— you can efficiently find common elements across multiple arrays with minimal overhead. Implement these strategies to refine your data manipulation capabilities, ensuring your code is efficient and adaptable to various complexities and needs.
No comments yet.