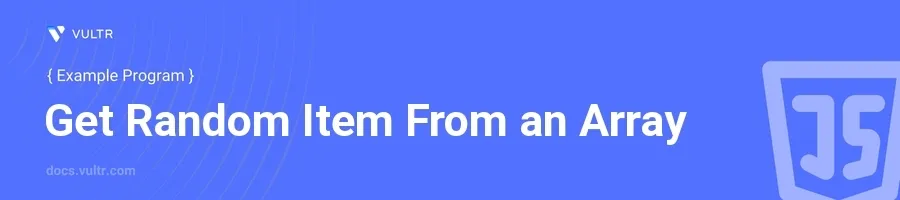
Introduction
Selecting a random item from an array is a common task in JavaScript, often performed in scenarios ranging from simple decision-making applications to complex data sampling in analytics. This capability can enhance the dynamism and user experience in web applications by introducing variability and unpredictability where needed.
In this article, you will learn how to effectively retrieve a random item from an array using JavaScript. The methods provided will guide you through various practical examples, ensuring you can implement these solutions in your web projects to handle different array structures and scenarios.
Basic Method to Fetch Random Item
Understanding the Random Selection Process
Appreciate the importance of the
Math.random()
function, which generates a random floating number between 0 and 1.Combine
Math.random()
withMath.floor()
to obtain an integer index. This index corresponds to a position in the array.javascriptfunction getRandomItem(arr) { const index = Math.floor(Math.random() * arr.length); return arr[index]; }
This function
getRandomItem
takes an arrayarr
as input and calculates a random index. It then returns the element at that index. TheMath.random()
function generates a number between 0 and 1, not including 1, and when multiplied by the length of the array, it's scaled to the array's range.Math.floor()
is then used to round down to the nearest whole number, ensuring the index is valid.
Example
Create an array of sample items.
Call the
getRandomItem
function to fetch a random element.javascriptconst foods = ['Apple', 'Banana', 'Cherry', 'Date', 'Elderberry']; const randomFood = getRandomItem(foods); console.log(randomFood);
In this example, the
getRandomItem
function selects a random food item from thefoods
array and logs it to the console. Every time you run the code, you can expect a different food item to be selected.
Enhanced Method with ES6 Features
Utilizing Destructuring and Arrow Functions
Understand the brevity and readability benefits of ES6 features.
Rewrite the random selection function using an arrow function and template literals for better clarity and efficiency.
javascriptconst getRandomItem = arr => arr[Math.floor(Math.random() * arr.length)];
This concise version uses an arrow function that directly returns the randomly selected item from the array passed as an argument. It omits the explicit return statement and brackets, adhering to ES6 syntax for simplicity.
Example Using ES6 Syntax
Define a new array with different types of elements.
Use the refactored
getRandomItem
function to pick a random element.javascriptconst elements = [10, 'Hello', true, { key: 'value' }, [1, 2, 3]]; const randomElement = getRandomItem(elements); console.log(randomElement);
The array
elements
contains multiple data types, demonstrating the function's versatility. The succinct ES6 version ofgetRandomItem
easily handles arrays containing various data types, fetching a random element each time.
Conclusion
Utilizing JavaScript to select a random item from an array is a useful skill that can be applied in numerous programming scenarios, from game development to data sampling. By implementing the methods discussed, you ensure your applications can generate variability and maintain user interest through randomness. Adapt these straightforward techniques to enhance features in your projects, leveraging both traditional and ES6 JavaScript syntax for maximum efficiency and code clarity.
No comments yet.