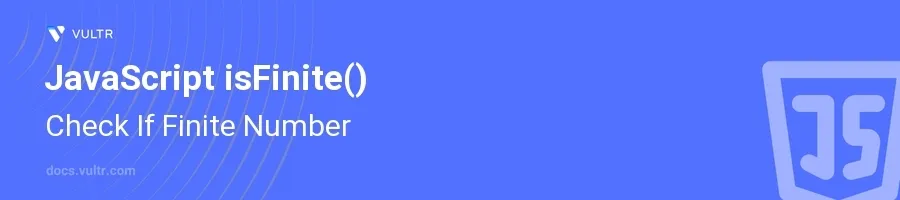
Introduction
The isFinite()
function in JavaScript is crucial when you need to determine if a given value is a finite number. This built-in function provides a straightforward approach to filter out infinite, NaN (Not a Number), or undefined values, which are common pitfalls in numerical computations and can lead to bugs if not handled correctly.
In this article, you will learn how to leverage the isFinite()
function in JavaScript. Get familiar with its basic usage, understand how it can be applied in different scenarios, and see how it differs from other similar functions like isNaN()
or when using type coercion.
Understanding isFinite()
Basic Usage of isFinite()
Check if a numeric value is finite.
javascriptvar result = isFinite(10); console.log(result); // Outputs: true
In this example,
10
is a finite number, and thusisFinite()
returnstrue
.
Edge Cases
Understand how non-numeric values are handled.
javascriptconsole.log(isFinite('Hello')); // Outputs: false console.log(isFinite('100')); // Outputs: true
In the first output,
isFinite()
returnsfalse
because 'Hello' is not a number. In the second output,isFinite()
implicitly converts the string '100' to the number 100, which is finite, hence it returnstrue
.
Dealing with Infinity and NaN
Discriminate between finite numbers and infinity or NaN values.
javascriptconsole.log(isFinite(Infinity)); // Outputs: false console.log(isFinite(-Infinity)); // Outputs: false console.log(isFinite(NaN)); // Outputs: false
Here,
Infinity
,-Infinity
, andNaN
are correctly identified as non-finite values.
Practical Usage Scenarios
Validating User Input as Numeric and Finite
Use
isFinite()
to ensure that user inputs are both numeric and limited to finite values.javascriptfunction validateInput(input) { return isFinite(input); } console.log(validateInput('500')); // Outputs: true console.log(validateInput('Infinity')); // Outputs: false console.log(validateInput('abc')); // Outputs: false
This function will return
true
only if the input is a finite number. This can prevent errors when calculations or numeric operations are performed later in your code.
Filtering Finite Numbers in Arrays
Employ
isFinite()
in an array filter operation to clean data.javascriptvar data = [5, Infinity, 0, NaN, 50]; var finiteData = data.filter(isFinite); console.log(finiteData); // Outputs: [5, 0, 50]
Here,
filter
utilizesisFinite()
to keep only the elements that are finite numbers from thedata
array, effectively cleaning the data.
Comparisons with Other Functions
Contrast
isFinite()
withNumber.isFinite()
and other type checking options.isFinite()
auto-converts the input to a number which can sometimes lead to unexpected results if a string that can be coerced to a number is passed.Number.isFinite()
does not convert types, thereby offering strict checking.
javascriptconsole.log(isFinite("42")); // Outputs: true console.log(Number.isFinite("42")); // Outputs: false
As shown,
isFinite()
returnstrue
when it coerces the string"42"
to the number42
, whereasNumber.isFinite()
does a strict check and returnsfalse
as the input is not a number.
Conclusion
The isFinite()
function in JavaScript is a straightforward yet powerful tool for validating whether a value is a finite number. It helps to ensure data integrity, especially when dealing with external inputs or data transformations in web applications. By mastering the usage of isFinite()
and understanding when to use it over or in conjunction with Number.isFinite()
, you enhance your ability to tackle common challenges in JavaScript involving numeric data. Implement this function mindful of implicit type coercion to ensure accurate validations and operations in your projects.
No comments yet.