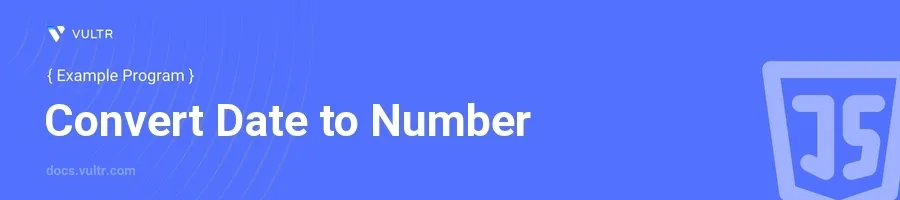
Introduction
The conversion of a date to a number in JavaScript is a common operation that is typically used to compare dates or to calculate time intervals efficiently. Numbers representing dates in JavaScript are based on the number of milliseconds since January 1, 1970, known as the Unix Epoch time.
In this article, you will learn how to convert a date to a number in JavaScript through various examples. You’ll understand how to utilize both built-in JavaScript functions and custom methods to perform these conversions effectively.
Convert Date to Number Using getTime()
Using the getTime()
Method
Create a Date object.
Utilize the
getTime()
method to convert the date to a numeric value representing the milliseconds since the Unix Epoch.javascriptconst date = new Date(); const milliseconds = date.getTime(); console.log(milliseconds);
This code snippet demonstrates converting the current date and time into milliseconds using the
getTime()
method. The printed value represents the time elapsed in milliseconds since January 1, 1970.
Assigning a Specific Date
Define a specific date by passing parameters to the
Date
constructor.Use
getTime()
to convert this date to a number.javascriptconst specificDate = new Date('December 17, 1995 03:24:00'); const specificMilliseconds = specificDate.getTime(); console.log(specificMilliseconds);
Here,
specificDate
is constructed to represent a specific point in time, andspecificMilliseconds
contains the numeric conversion of this date. Such conversions are helpful for setting time-based conditions or storing date values efficiently.
Convert Date to Number Using Unary Plus Operator
Using the Unary Plus Operator
Create a Date object.
Apply the unary plus (
+
) operator to convert the date to a number directly.javascriptconst dateNow = new Date(); const dateNumber = +dateNow; console.log(dateNumber);
Using the unary plus before a Date object directs JavaScript to convert the date into its numeric representation automatically. This method is shorter and often used in scenarios where code brevity is preferred.
Convert Date to Number Using valueOf()
Utilizing valueOf()
Method
Initialize a Date object.
Call the
valueOf()
method on the date to obtain its numeric form.javascriptconst today = new Date(); const numericToday = today.valueOf(); console.log(numericToday);
The
valueOf()
method in JavaScript Date objects returns the primitive value of the specified object as a number. In this example,numericToday
reflects the number of milliseconds since the Unix Epoch, similar to thegetTime()
method, but it’s often used in an object-oriented context.
Conclusion
Converting dates to numbers in JavaScript enhances the flexibility and efficiency of handling date and time data, particularly in comparisons and calculations. Whether using the getTime()
, unary plus, or valueOf()
method, each provides a robust way to convert JavaScript Date objects into a numerical format. Master these techniques to manage date-time values more effectively in your JavaScript applications, maintaining precision and improving performance for time-sensitive operations.
No comments yet.