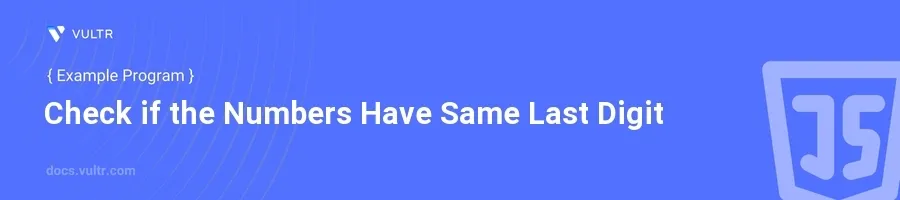
Introduction
Comparing the last digits of numbers can be a frequent requirement in various programming tasks, such as numerical validations or matching specific criteria in a data set. In JavaScript, this kind of operation is straightforward thanks to the language's flexibility in handling numbers and strings.
In this article, you will learn how to write a JavaScript function to check if two or more numbers have the same last digit. Explore practical examples that demonstrate how to implement and utilize this function effectively in different scenarios.
Writing the Basic Function
Define the Function
Start by creating a function named
haveSameLastDigit
. This function will accept an arbitrary number of arguments.Inside the function, use the
Array.prototype.every
method to ensure every number meets a specific condition.Convert each number to a string and compare the last character of these strings.
javascriptfunction haveSameLastDigit(...numbers) { return numbers.every((num, _, arr) => num.toString().slice(-1) === arr[0].toString().slice(-1)); }
In this code,
...numbers
uses the rest parameter syntax to gather any number of arguments into an array. Theevery
method checks whether all numbers have the same last digit as the first number in the input array.
Example Usage of the Basic Function
Test the function with numbers that have the same last digit.
Test the function with numbers with different last digits.
javascriptconsole.log(haveSameLastDigit(27, 537, 17)); // true console.log(haveSameLastDigit(234, 78, 19)); // false
These examples show how the function is used to check the last digit of provided numbers and returns
true
if they all match, andfalse
otherwise.
Extending the Functionality
Modify Function to Handle Edge Cases
Consider adding input validation to ensure the function handles different data types and edge cases gracefully.
Return
false
if input arguments are not numbers or the array is empty.javascriptfunction haveSameLastDigit(...numbers) { if (numbers.length === 0 || numbers.some(num => typeof num !== 'number')) { return false; } return numbers.every((num, _, arr) => num.toString().slice(-1) === arr[0].toString().slice(-1)); }
The updated function now checks for the correct data type and ensures that it does not proceed with comparisons if there's invalid input or no numbers at all.
Handle Numeral Systems Beyond Decimal
Adapt the function to work with numbers in different bases, such as hexadecimal or binary.
Allow an additional parameter specifying the base, and adjust the string conversion accordingly.
javascriptfunction haveSameLastDigit(base = 10, ...numbers) { if (numbers.length === 0 || numbers.some(num => typeof num !== 'number')) { return false; } return numbers.every((num, _, arr) => num.toString(base).slice(-1) === arr[0].toString(base).slice(-1)); }
Here, an optional
base
parameter has been added. The function now converts numbers into strings according to the specified base before comparing their last digits.
Conclusion
The ability to check if numbers share the same last digit in JavaScript is a useful skill, especially in data validation or when processing different numeral systems. The examples provided illustrate a flexible approach to achieving this, allowing for a better understanding of string manipulation and condition checking in JavaScript. By customizing this function, you ensure that it can adapt to various requirements and input types, making your JavaScript code more robust and adaptable to changing needs.
No comments yet.