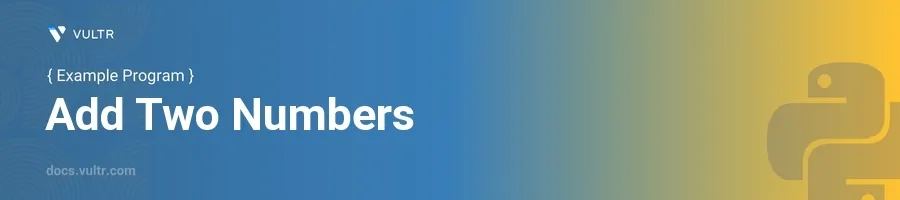
Introduction
Adding two numbers is a fundamental task in any programming language, serving as a common starting point for new learners to understand syntax and operations. Python, with its clean and straightforward syntax, offers a user-friendly way to handle basic arithmetic operations like addition.
In this article, you will learn how to add two numbers using Python. The discussion includes examples using literals, variables, and user inputs, illustrating different scenarios where number addition becomes necessary.
Adding Numbers Using Literals
Direct Addition of Two Numbers
Open your Python editor or an interactive shell.
Type the direct addition of two values.
pythonresult = 3 + 2 print(result)
The code above directly adds the numbers 3 and 2, storing the result in the variable
result
. Theprint()
function then outputs the result, which is 5.
Adding Numbers Stored in Variables
Basic Variable Addition
Define variables to store your numbers.
Perform addition using these variables.
pythonnum1 = 5 num2 = 7 sum = num1 + num2 print(sum)
This example stores the numbers 5 and 7 in
num1
andnum2
respectively. The addition is performed, and the result (12) is stored in the variablesum
, which is then printed.
Using User Input to Add Numbers
Addition of Two Numbers from User Input
Use the
input()
function to gather numbers from the user.Convert the string inputs to integers.
Add the numbers and display the result.
pythonfirst_number = int(input("Enter the first number: ")) second_number = int(input("Enter the second number: ")) total = first_number + second_number print("The sum of the numbers is:", total)
In the script above,
input()
collects string data from the user, which is then converted to integers usingint()
. The integers are added together and the sum is displayed. This method allows dynamic input from users at runtime.
Conclusion
Python provides a simple and efficient framework for performing basic arithmetic operations such as adding two numbers. Whether you're dealing with hardcoded values, variables, or user inputs, Python's easy-to-understand syntax makes these tasks straightforward. Following these examples, enable yourself to handle basic number manipulation in your applications, paving the way for more complex programming tasks.
No comments yet.