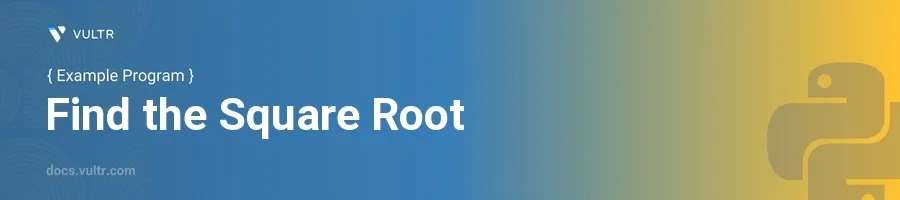
Introduction
Computing the square root of a number is a frequent requirement in many mathematical computations. In Python, this operation can be performed in several ways, including using built-in functions and applying specific algorithms. The square root of a number, "n", is the number that, when multiplied by itself, gives "n". It is often denoted as √n.
In this article, you will learn how to implement different methods to find the square root in Python. You'll explore the use of the math
module, handling perfect and non-perfect squares, and implementing custom functions for educational purposes.
Using the math
Module
The math
module in Python provides a collection of mathematical functions, including sqrt()
, which efficiently computes the square root of a number.
Calculate Square Root of Perfect Squares
Import the
math
library.Use the
sqrt()
function to compute the square root.pythonimport math number = 16 square_root = math.sqrt(number) print("The square root of", number, "is", square_root)
This code computes the square root of 16. Here,
math.sqrt()
directly calculates the square root and the output will be 4.0.
Handling Non-Perfect Squares
Understand that non-perfect squares will have a square root that is a floating-point number.
Use the same
sqrt()
function for non-perfect squares.pythonimport math number = 17 square_root = math.sqrt(number) print("The square root of", number, "is", square_root)
For the non-perfect square 17, the code calculates the square root and displays it as a floating-point number.
Custom Function for Square Root
For educational and deeper understanding, implementing a custom function to compute the square root can be quite informative. One common method uses the Newton-Raphson iteration.
Create a Custom Square Root Function
Define a function that uses the Newton-Raphson method to compute the square root.
Ensure the function iteratively approaches the square root.
pythondef custom_sqrt(n, precision=0.00001): guess = n while abs(guess * guess - n) > precision: guess = (guess + n / guess) / 2 return guess number = 23 result = custom_sqrt(number) print("The square root of", number, "is approximately", result)
This function starts with an initial guess that the square root might be the number itself (
n
). It iteratively improves the guess based on the Newton-Raphson method until the error is within the specified precision.
Conclusion
Calculating the square root in Python can be approached in multiple ways. Using the math
module provides a quick and efficient method suitable for many practical applications. For deeper understanding or custom requirements, implementing a custom function like the one using the Newton-Raphson method offers flexibility and control over the computation process. Use these methods as needed to enhance your numerical computing tasks, ensuring clarity and precision in your mathematical operations.
No comments yet.