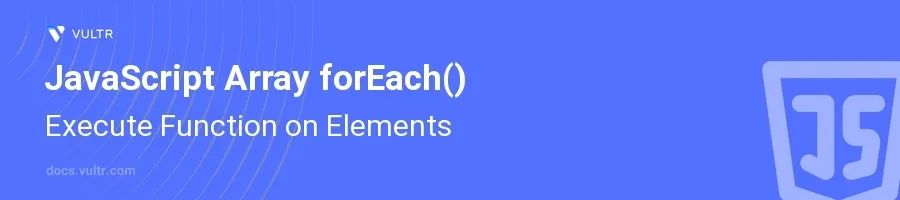
Introduction
The JavaScript forEach()
method is an integral part of the Array prototype that allows for executing a function on each element in an array. This method simplifies the process of iterating over array elements, eliminating the need for traditional loop constructs like for
or while
, thereby making the code more readable and concise.
In this article, you will learn how to use the JavaScript forEach()
method to iterate over array elements effectively. Explore practical examples of forEach()
for array manipulation, working with functions, and handling asynchronous operations in JavaScript.
Basic Usage of forEach() Method in JavaScript
Execute a Simple Function on Each Item
Define an array of items.
Use the
forEach()
method to apply a function to each element.javascriptconst fruits = ['apple', 'banana', 'cherry']; fruits.forEach(function(item, index) { console.log(index, item); });
This code prints each fruit in the array along with its index to the console. The
forEach()
method takes a callback function as an argument, which is applied to every element of the array.
Modify Array Elements
Start with a numeric array.
Use the
forEach()
method to modify each element.javascriptconst numbers = [1, 2, 3]; numbers.forEach((value, index, arr) => { arr[index] = value * 10; }); console.log(numbers);
Here, each number in the array
numbers
is multiplied by 10. TheforEach()
method provides access not just to the array element and its index, but also to the array itself, allowing direct modification.
Advanced Usage of JavaScript forEach() Method
Using forEach() with Object Arrays
Create an array of objects.
Use
forEach()
to iterate over the objects and perform operations.javascriptconst users = [{ name: 'Alice', age: 25 }, { name: 'Bob', age: 30 }]; users.forEach(user => { console.log(`${user.name} is ${user.age} years old.`); });
In this snippet,
forEach()
is used to log details about each user. This example demonstrates the utility offorEach()
in handling arrays of objects for tasks such as generating reports or processing data.
Handling Asynchronous Logic with JavaScript forEach() Method
Understand that
forEach()
is not suitable for handling promises directly due to its synchronous nature.Use
Promise.all
withmap()
for asynchronous operations instead.javascriptconst urls = ['url1', 'url2', 'url3']; const fetchPromises = urls.map(url => fetch(url)); Promise.all(fetchPromises) .then(responses => responses.forEach(response => console.log(response.status))) .catch(error => console.error('Failed to fetch:', error));
Here, for handling asynchronous operations,
map()
is used to create an array of Promises, and thenPromise.all()
is used to wait for all the Promises to resolve. After all promises resolve,forEach()
is employed to handle each response effectively.
Common Mistakes with JavaScript forEach()
Using return
, break
, or continue
Incorrectly
Understand that
forEach()
does not supportbreak
,continue
, or earlyreturn
in the loop.These statements only exit the callback function, not the entire loop.
javascriptconst items = [1, 2, 3, 4]; items.forEach(item => { if (item === 3) return; // Only skips this iteration console.log(item); });
In this example,
3
is skipped, but the loop continues. To exit a loop early or skip iterations like in a traditionalfor
loop, consider usingfor
orfor...of
.
Using await
Inside forEach
Know that
forEach()
is synchronous and does not wait forawait
to resolve.Asynchronous logic inside
forEach()
will not execute in the expected sequence.javascriptconst asyncTask = async (item) => { await new Promise(resolve => setTimeout(resolve, 1000)); console.log(item); }; const items = [1, 2, 3]; items.forEach(async (item) => { await asyncTask(item); });
This code runs all tasks in parallel, not sequentially. To run async operations in order, use a
for...of
loop withawait
.
Conclusion
The forEach()
method in JavaScript is a powerful tool for iterating over array elements and performing actions on them. It simplifies code and enhances readability by removing explicit loops and providing direct access to each element. Whether dealing with simple arrays or complex data structures, forEach()
helps maintain clean and effective code. Utilize this method to streamline your array operations and improve the maintainability of your JavaScript code.
No comments yet.