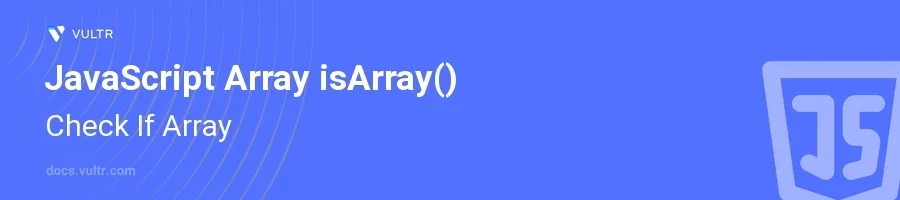
Introduction
The isArray()
method in JavaScript determines whether a given value is an array. This method is crucial because arrays are one of the key data structures in JavaScript, used for a multitude of applications ranging from data handling to manipulation tasks. Its reliable check ensures our code logic remains robust and error-free when operations specific to arrays need to be performed.
In this article, you will learn how to effectively use the isArray()
method to ascertain whether a given value conforms to an array type. Explore practical examples showcasing its implementation to help you understand its utility and integration in various programming scenarios.
Understanding isArray() Method
Basic Usage of isArray()
Import the
Array
object from JavaScript which provides theisArray()
function.Pass the variable you want to check into
Array.isArray()
method.javascriptlet arr = [1, 2, 3]; let result = Array.isArray(arr); console.log(result); // Output: true
This example demonstrates how to check if
arr
is an array. The function returnstrue
asarr
is indeed an array.
Check Non-Array Values
Realize that
Array.isArray()
will returnfalse
for values that are not arrays.Apply the check to several types of values to see the results.
javascriptconsole.log(Array.isArray({})); // Output: false console.log(Array.isArray(123)); // Output: false console.log(Array.isArray('Array')); // Output: false console.log(Array.isArray(undefined)); // Output: false
These are various non-array data types checked against
Array.isArray()
. As none of these are arrays, it correctly returnsfalse
for each.
Dealing with Array-like Objects
Understand that array-like objects (such as NodeLists or arguments object) are not considered arrays by the
isArray()
method.Check the array-like objects to clarify this distinction.
javascriptfunction testArguments() { return Array.isArray(arguments); } console.log(testArguments(1, 2, 3)); // Output: false
Here, even though
arguments
resembles an array and contains elements that can be accessed by indices,Array.isArray()
identifies it as not being an array.
Conclusion
The Array.isArray()
function is a dependable method to ascertain if a value is an actual array or not in JavaScript. This method is crucial for maintaining the integrity of operations and functionalities that specifically deal with array manipulations. By mastering isArray()
, you prevent potential bugs that might occur from misidentified data types and ensure that your code remains versatile and robust. Implement this check regularly in your scripts to uphold high standards in data handling and processing.
No comments yet.