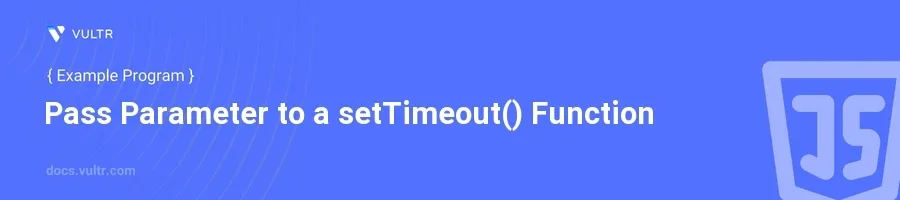
Introduction
The setTimeout()
function in JavaScript is used to execute a block of code or a specific function after a specified delay. This function is incredibly versatile in managing operations that require a timing mechanism, such as animations, API calls, or any operation that needs to be delayed. It's fundamental in handling time-based logic in web applications.
In this article, you will learn how to pass parameters to a setTimeout()
function in JavaScript. This technique enhances the function's versatility, allowing dynamic actions based on variable inputs. Detailed examples will guide you through different methods of passing parameters effectively.
Passing Parameters Directly in the setTimeout() Call
Passing Single Parameter
Define the function that will be executed after a delay.
Set up the
setTimeout()
function and pass the parameter directly by placing it after the delay argument.javascriptfunction greet(name) { console.log("Hello, " + name + "!"); } setTimeout(greet, 2000, 'Alice');
In this code, the
greet
function expects one parameter,name
. WithsetTimeout()
, the function is scheduled to execute after 2 seconds, passing 'Alice' as the argument. When the timer elapses, it outputs:Hello, Alice!
.
Passing Multiple Parameters
Prepare a function that can handle multiple parameters.
Configure
setTimeout()
to pass multiple parameters after the delay.javascriptfunction displayUserDetails(name, age) { console.log(`User ${name} is ${age} years old.`); } setTimeout(displayUserDetails, 1500, 'Bob', 25);
Here, the
displayUserDetails
function requires two parameters. This configuration outputsUser Bob is 25 years old.
after a 1.5 seconds delay.
Using Closures to Pass Parameters
Closure with an Anonymous Function
Capture parameters using a closure inside an anonymous function.
Use this function as the argument to
setTimeout()
.javascriptconst greeting = 'Hello'; const user = 'Charlie'; setTimeout(function() { console.log(greeting + ", " + user + "!"); }, 1000);
The closure created by the anonymous function inside
setTimeout()
captures 'greeting' and 'user' from its surrounding scope. After a delay of 1 second, it will execute and print:Hello, Charlie!
.
Closure with External Function
Define a function that returns another function, creating a closure that captures external parameters.
Use the returned function for the
setTimeout()
call.javascriptfunction setupAlert(message) { return function() { alert(message); }; } setTimeout(setupAlert('You have a new message!'), 3000);
setupAlert()
returns a function that encloses themessage
parameter. The external function is executed after 3 seconds, showing an alert dialog with the message.
Using ES6 Arrow Functions for Simplicity
Simple Usage with Arrow Functions
Employ an arrow function to directly embed parameters into the
setTimeout()
.This method provides a concise and clean way to implement timeouts with parameters.
javascriptconst item = 'Ice Cream'; setTimeout(() => console.log("Do you like " + item + "?"), 2500);
The arrow function cleanly encapsulates the parameter within its lexical scope, displaying
Do you like Ice Cream?
after 2.5 seconds.
Conclusion
Getting comfortable with passing parameters to the setTimeout()
function in JavaScript allows for more dynamic and timed interactions in your applications. Whether you're scheduling single or multiple parameters, using closures, or leveraging the simplicity of ES6 arrow functions, these techniques provide powerful tools to create responsive, time-based code functionalities. Adapt these examples to fit the context of your projects and harness the full potential of JavaScript's setTimeout()
for effective time-based programming.
No comments yet.