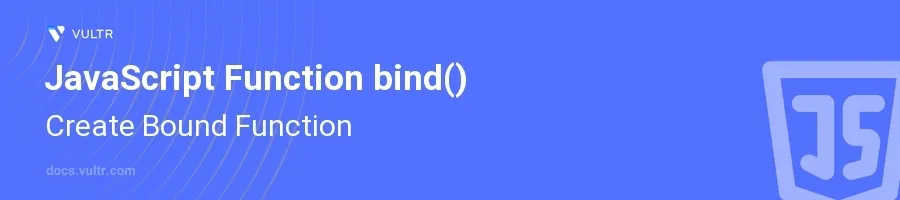
Introduction
The bind()
method in JavaScript is a powerful function that allows you to set the this
value for one or more functions. This method is particularly useful for handling the this
context in asynchronous callbacks and when passing function references around in object-oriented programming.
In this article, you will learn how to effectively use the bind()
method to maintain the context of this
across various scenarios. Explore practical applications of bind()
including event handling and with setTimeout, showcasing how it ensures functions operate with the intended context.
Understanding bind() in JavaScript
Basic Usage of bind()
The bind()
method creates a new function that, when called, has its this
keyword set to the provided value. Here's how to create a bound function:
Define a function where
this
is significant.Use
bind()
to create a new function with a specifiedthis
value.javascriptconst module = { x: 42, getX: function () { return this.x; } }; const unboundGetX = module.getX; console.log(unboundGetX()); // undefined because `this` is not bound const boundGetX = unboundGetX.bind(module); console.log(boundGetX()); // 42, `this` is now bound to `module`
This example demonstrates how
bind()
resolves the common problem where functions lose their intendedthis
context when assigned or passed around as callbacks.
Using bind() with Parameters
bind()
can also predefine initial parameters for the function being bound. This is useful for situations where you want to preset some arguments.
Define a function that accepts several arguments.
Use
bind()
to pre-set some of these arguments.javascriptfunction addNumbers(a, b) { return a + b; } const addFive = addNumbers.bind(null, 5); console.log(addFive(10)); // 15
Here,
addNumbers
is bound with the first parameter set to 5. The functionaddFive
only needs one additional parameter to complete its operation.
Practical Applications of bind()
Event Handling
When adding event listeners in JavaScript, the this
context can unwittingly change, especially if the method belongs to a class or object.
Consider a class with a method used as an event handler.
Bind the method to
this
in the constructor to maintain context.javascriptclass Button { constructor(value) { this.value = value; this.clickHandler = this.clickHandler.bind(this); } clickHandler() { alert(this.value); } } const button = new Button('Hello'); document.querySelector('button').addEventListener('click', button.clickHandler);
Binding the
clickHandler
method ensures it always has the correctthis
, corresponding to the instance ofButton
even when triggered by an event listener.
Handling setTimeout
and setInterval
Using setTimeout
or setInterval
can also lead to loss of context. Binding the function keeps this
aligned with the intended object.
Create an object method that you want to run later with
setTimeout
.Bind the object method before passing it to
setTimeout
.javascriptconst person = { name: 'Jane Doe', greet: function() { console.log(`Hello, ${this.name}`); } }; setTimeout(person.greet.bind(person), 1000);
Using
bind()
in this way ensures thatgreet
maintains the person object as its context, even when executed asynchronously throughsetTimeout
.
Conclusion
Utilizing the bind()
method in JavaScript enables you to control the execution context of functions, providing reliability especially in scenarios involving callbacks and asynchronous programming. This method proves invaluable in object-oriented patterns and event-driven programming, among other use cases. Mastering bind()
enhances the robustness and reusability of your JavaScript code, ensuring that functions execute with predictable behavior in various contexts.
No comments yet.