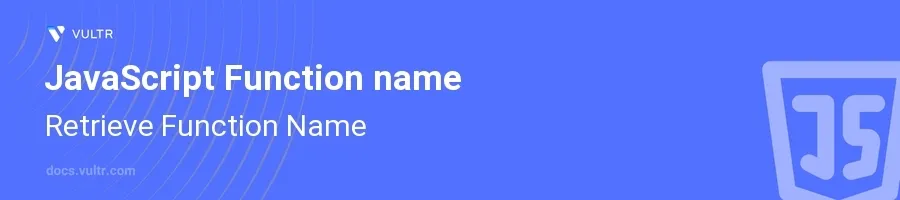
Introduction
In JavaScript, functions are objects, and like many other objects, they have properties. One useful property is name
, which holds the name of the function. This attribute can be particularly handy in debugging, when you want to know the name of the function currently being executed, or when you're working with higher-order functions and need to trace them better in your application.
In this article, you will learn how to retrieve the name of a function using the name
property in JavaScript. Explore practical examples to understand how this feature behaves across different types of functions including regular functions, anonymous functions, and functions assigned to variables.
Function Name Basics
Retrieve Function Name of a Regular Function
Retrieving the name of a standard function in JavaScript is straightforward. Here’s how to do it:
Define a regular named function.
Access its
name
property.javascriptfunction exampleFunction() {} console.log(exampleFunction.name); // Output: "exampleFunction"
This code defines a function named
exampleFunction
and retrieves its name usingexampleFunction.name
, which outputs the string"exampleFunction"
.
Working with Variable-Assigned Functions
When functions are assigned to variables, the name
property can still be used to ascertain the function's name. Here's how:
Create a function and assign it to a variable.
Print the function's
name
property.javascriptconst myFunc = function demoFunction() {}; console.log(myFunc.name); // Output: "demoFunction"
Here, the function is anonymously assigned to
myFunc
but originally defined asdemoFunction
. By accessingmyFunc.name
, JavaScript returns the original function name"demoFunction"
.
Working with Arrow Functions
Arrow functions behave a little differently when it comes to the name
property, especially when they are anonymous:
Define an arrow function assigned to a variable.
Retrieve the name of the function.
javascriptconst arrowFunction = () => {}; console.log(arrowFunction.name); // Output: "arrowFunction"
In modern JavaScript environments, like latest versions of Node.js and browsers, the
name
property can derive the variable name to which the arrow function was assigned, outputting here"arrowFunction"
.
Anonymous Functions and Default Naming
Anonymous functions typically don’t have a name, but the context in which they are used can influence the output of the name
property:
Define an anonymous function assigned to a variable.
Check the function's
name
property.javascriptconst anonymousFunc = function() {}; console.log(anonymousFunc.name); // Output: "anonymousFunc" (in modern environments)
Depending on the environment, older versions might simply return an empty string for anonymous functions, while newer JavaScript engines give the variable name as the function name.
Special Cases and Exceptions
Functions as Object Methods
When functions are defined as object methods, you can still access their names similarly:
Define a function as a method of an object.
Use the
name
property to retrieve the function name.javascriptconst obj = { myMethod: function() {} }; console.log(obj.myMethod.name); // Output: "myMethod"
This method within the object
obj
inherits its key as its name.
Conclusion
The name
property of JavaScript functions provides a helpful way to identify functions, which is incredibly useful for debugging and logging purposes. Whether you're working with traditional functions, functions assigned to variables, or arrow functions, understanding how to use this property enhances your ability to manage and debug your code. Remember to consider how different JavaScript engines might treat anonymity and naming to ensure compatibility and expected behavior across different execution environments.
No comments yet.