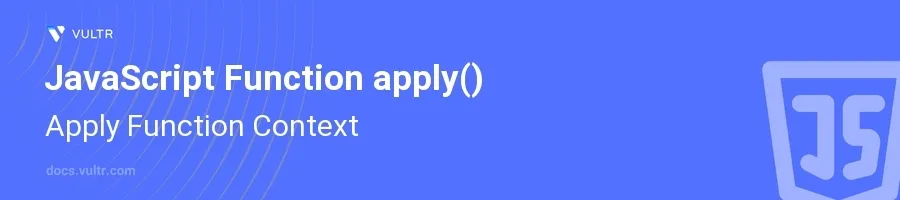
Introduction
The apply()
method in JavaScript is a powerful function that you can use to call another function, while explicitly setting the this
context and passing arguments as an array. This method is particularly useful in object-oriented programming when the context of this
needs to be controlled, and when a function needs to be invoked with a dynamically determined set of arguments.
In this article, you will learn how to use the apply()
method effectively to manage function contexts and arguments in JavaScript. Discover practical applications of apply()
, including inheriting constructors, working with array-like objects, and more.
Understanding the apply() Method
Basic Usage of apply()
Recognize that
apply()
allows you to execute a function with a specifiedthis
value.Pass arguments to the function as an array or an array-like object.
javascriptfunction greet(name, city) { console.log(`Hello, ${name} from ${city}!`); } const person = { name: 'John', city: 'New York' }; greet.apply(person, [person.name, person.city]);
This example uses
apply()
to invoke thegreet
function, settingthis
to theperson
object and passing the parameters as an array.
Manipulating Array-like Objects
Use
apply()
in combination with Math functions to process values in array-like objects.Smoothly convert an array-like object to an array if necessary.
javascriptconst scores = { '0': 98, '1': 95, '2': 93, 'length': 3 // Length is necessary for array-like objects }; const maxScore = Math.max.apply(null, Array.prototype.slice.call(scores)); console.log(`Highest score: ${maxScore}`);
In this snippet,
apply()
allowsMath.max
to takescores
as an array-like object, calculating the maximum value.
Using apply() with Constructor Chaining
Apply the
apply()
method to carry out constructor chaining, where one constructor calls another constructor within the same object.javascriptfunction Product(name, price) { this.name = name; this.price = price; } function Food(name, price) { Product.apply(this, [name, price]); this.category = 'food'; } var cheese = new Food('feta', 5); console.log(cheese);
In this example,
Food
inherits properties fromProduct
by callingProduct.apply()
. Here,this
refers to theFood
instance.
Advanced Techniques Using apply()
Spreading Elements
Utilize
apply()
to spread elements in an iterable object.javascriptlet numbers = [9, 4, 7, 1]; let maxNum = Math.max.apply(null, numbers); console.log(`Maximum number: ${maxNum}`);
In this case,
apply()
is used to spread thenumbers
array into individual arguments forMath.max()
.
Binding Functions
Recognize that
apply()
can be used to bind a function to an object dynamically, similar to usingbind()
, but invoked instantaneously.javascriptlet runner = { speed: 10, run: function() { console.log(`Running at ${this.speed} km/h`); } }; setTimeout(function() { runner.run.apply(runner); }, 1000);
This script sets up a delayed action to call the
run
function, explicitly settingthis
to reference therunner
object.
Conclusion
The apply()
method in JavaScript is an excellent tool for flexible function invocation, allowing for specific handling of the this
context and argument passing. By leveraging apply()
, you expand your capacity to build more adaptable and maintainable JavaScript code, whether it’s through function binding, constructor chaining, or working with array-like objects. Embrace these techniques to enhance your coding strategies and optimize your application's performance.
No comments yet.