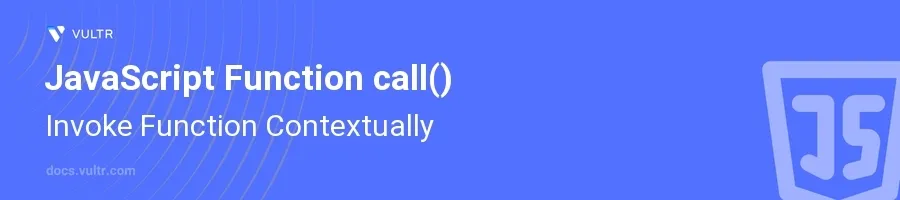
Introduction
The call()
method in JavaScript is a powerful tool for manipulating the context of a function call, enabling developers to specify the this
value and pass arguments to the called function as needed. This method is particularly useful in object-oriented programming, where the context in which a function operates is crucial for correct behavior and data manipulation.
In this article, you will learn how to use the call()
method to control the execution context of functions in JavaScript. Explore various examples that demonstrate how call()
can be employed in different scenarios to enhance code modularity and reusability.
Understanding the call() Method
Basic Usage of call()
Define a function that uses
this
.Use
call()
to invoke the function with a specified context.javascriptfunction showDetails() { console.log(`Name: ${this.name}, Age: ${this.age}`); } const person = { name: 'Alice', age: 30 }; showDetails.call(person);
This snippet demonstrates how
call()
changes the context ofshowDetails()
to theperson
object, enabling access to its properties within the function.
Passing Arguments with call()
Modify a function to accept more parameters.
Specify additional arguments in
call()
after the context.javascriptfunction updateDetails(job, city) { console.log(`Name: ${this.name}, Age: ${this.age}, Job: ${job}, City: ${city}`); } updateDetails.call(person, 'Developer', 'New York');
In this example,
call()
not only sets the function'sthis
to theperson
object but also passesjob
andcity
as arguments toupdateDetails()
.
Using call() for Method Borrowing
Borrow Methods from Different Objects
Identify a method in one object that should be used on another.
Use
call()
to borrow that method.javascriptconst person1 = { fullName: function() { return `${this.firstName} ${this.lastName}`; } }; const person2 = { firstName: 'John', lastName: 'Doe' }; console.log(person1.fullName.call(person2));
Here,
person1
'sfullName()
method is applied toperson2
viacall()
, showing how methods can be borrowed and reused across different object instances.
Handling Arrays as Function Arguments
Use call() to Apply Array Methods
Consider a scenario where array-like objects need array methods.
Apply an array method using
call()
.javascriptfunction listArguments() { const args = Array.prototype.slice.call(arguments); console.log(args); } listArguments(1, 'blue', false);
This code segment uses
call()
to convertarguments
, an array-like object, into a true array, demonstratingcall()
's flexibility in handling JavaScript's built-in objects and methods.
Conclusion
The call()
function in JavaScript offers a dynamic approach to function invocation by allowing the customization of the this
context and the passing of arguments. Its utility in method borrowing and handling array-like objects enables developers to write cleaner, more modular code. Through practical applications and understanding of call()
, improve code efficiency and maintainability in a variety of programming situations. Embrace these techniques to fine-tune your functions' behavior based on the context required.
No comments yet.