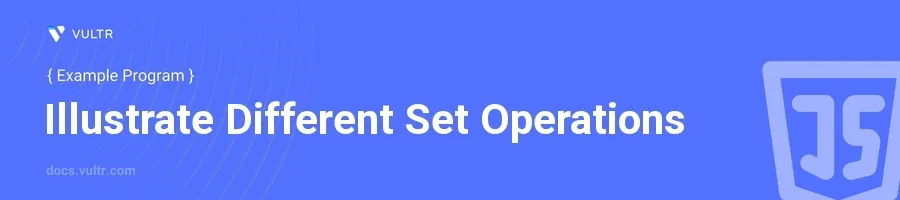
Introduction
The Set object in JavaScript allows you to store unique values of any type, whether primitive values or object references. It is especially handy in situations requiring uniqueness among the elements stored, promoting both efficiency and ease of data manipulation. The fact that all values in a Set are unique simplifies many common tasks in programming, such as removing duplicates from an array or performing various set operations like union, intersection, and difference.
In this article, you will learn how to use the Set object in JavaScript to perform a range of set operations. Explore detailed examples that demonstrate how to implement union, intersection, difference, and subset checks effectively using JavaScript. Each section is accompanied by code snippets and explanations to ensure you can integrate these patterns into your projects seamlessly.
Basic Set Operations in JavaScript
Creating a Set and Adding Elements
Initialize a new Set.
Add elements to the Set using the
add
method.javascriptconst fruits = new Set(); fruits.add("apple"); fruits.add("banana"); fruits.add("orange");
This code snippet creates a Set named
fruits
and adds three elements to it: "apple", "banana", and "orange".
Checking for Element Existence
Use the
has
method to check if an element is in the Set.javascriptconsole.log(fruits.has("banana")); // outputs: true console.log(fruits.has("grape")); // outputs: false
The
has
method confirms whether "banana" is present, returningtrue
, and checks "grape", which is not in the Set, thus returningfalse
.
Advanced Set Operations
Performing a Union of Two Sets
Define two sets with different elements.
Use the spread operator to combine them into a new Set, effectively performing the union.
javascriptconst setA = new Set([1, 2, 3]); const setB = new Set([3, 4, 5]); const union = new Set([...setA, ...setB]); console.log(union); // outputs: Set(5) {1, 2, 3, 4, 5}
This merges
setA
andsetB
, where the resulting Set includes all elements from both contributing sets, demonstrating the union operation.
Finding the Intersection of Two Sets
Identify elements common to both sets by filtering one Set based on the contents of the other.
javascriptconst intersection = new Set([...setA].filter(x => setB.has(x))); console.log(intersection); // outputs: Set(1) {3}
Here, filtering through
setA
with the condition that elements must also exist insetB
gives the intersection, which contains only the element3
.
Calculating the Difference between Two Sets
Use filtering to find elements in one Set that are not in another.
javascriptconst difference = new Set([...setA].filter(x => !setB.has(x))); console.log(difference); // outputs: Set(2) {1, 2}
The difference operation here yields a Set of elements that are in
setA
but not insetB
, namely1
and2
.
Checking if One Set is a Subset of Another
Determine if all elements of one Set exist within another Set.
javascriptconst isSubset = [...setA].every(element => setB.has(element)); console.log(isSubset); // outputs: false
This operation checks if every element in
setA
is also insetB
. Since not all are, it returnsfalse
, indicatingsetA
is not a subset ofsetB
.
Conclusion
Set operations in JavaScript are powerful tools that help manage collections of data effectively and efficiently. By utilizing the Set object, you can ensure uniqueness and easily perform standard set operations like union, intersection, difference, and checking subsets. The techniques discussed here are fundamental for data manipulation, allowing you to handle large datasets and complex data structures effortlessly. Integrate these operations in your JavaScript projects to simplify and enhance your code management and data integrity.
No comments yet.