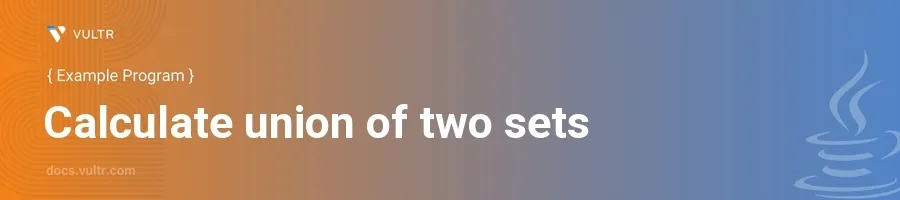
Introduction
Calculating the union of two sets is a common task in computer applications, especially when dealing with data analysis or algorithms that require set operations. The union operation combines all the elements from two sets without duplicating any items. Java, with its robust Collection Framework, provides various ways to perform this operation efficiently.
In this article, you will learn how to calculate the union of two sets using Java. You'll see examples using different Java collection classes and methods so you can choose the best approach based on your specific requirements.
Calculating Union Using HashSet
Basic Union Operation
Initialize two sets with the values you want to combine.
Create a new
HashSet
to store the union.Add all elements from both sets into the union set using the
addAll()
method.javaSet<Integer> setA = new HashSet<>(Arrays.asList(1, 2, 3, 4)); Set<Integer> setB = new HashSet<>(Arrays.asList(3, 4, 5, 6)); Set<Integer> union = new HashSet<>(setA); union.addAll(setB); System.out.println(union);
In this example,
setA
andsetB
are combined intounion
. TheaddAll()
method ensures that all elements from both sets are added tounion
, and since it's a set, it automatically discards any duplicates. The output will be[1, 2, 3, 4, 5, 6]
.
Considerations with Different Data Types
Recognize that the union operation is not limited to integers or a single data type.
Apply the union operation with
HashSet
on various data types.javaSet<String> setA = new HashSet<>(Arrays.asList("apple", "banana")); Set<String> setB = new HashSet<>(Arrays.asList("banana", "cherry")); Set<String> union = new HashSet<>(setA); union.addAll(setB); System.out.println(union);
The
union
ofsetA
andsetB
includes three different fruit names. As before, duplicates are removed, yielding the output["apple", "banana", "cherry"]
.
Calculating Union Using Stream API
Stream API for Union
Utilize Java 8's Stream API for a more modern approach.
Merge two sets and collect the results into a new set.
javaSet<Integer> setA = new HashSet<>(Arrays.asList(1, 2, 3, 4)); Set<Integer> setB = new HashSet<>(Arrays.asList(3, 4, 5, 6)); Set<Integer> union = Stream.concat(setA.stream(), setB.stream()) .collect(Collectors.toSet()); System.out.println(union);
This approach uses
Stream.concat()
to form a combined stream of elements from both sets, which is then collected into a newHashSet
. The result is similar to the previous examples but leverages the functional programming capabilities of Java Streams.
Stream API with Custom Objects
Define a custom class for more complex data types.
Use the Stream API to handle objects from this custom class.
javaclass CustomObject { String key; int value; // Constructor, getters, setters, and hashCode/equals methods omitted for brevity } Set<CustomObject> setA = new HashSet<>(); Set<CustomObject> setB = new HashSet<>(); // Assume objects are added to sets here Set<CustomObject> union = Stream.concat(setA.stream(), setB.stream()) .collect(Collectors.toSet()); System.out.println(union);
When using custom objects, ensure that
hashCode()
andequals()
methods are properly overridden in your class to accurately identify duplicates in set operations.
Conclusion
Calculating the union of two sets in Java can be done efficiently using either HashSet
with the addAll()
method or the Stream API with collect()
. The method you choose will depend on your specific context, such as the complexity of the data type and personal or project-based preference for procedural versus functional programming style. Both methods ensure your sets are combined effectively, maintaining the integrity of set properties by automatically filtering out duplicates. Expand on these examples to tailor set operations to your needs and leverage Java's powerful data handling capabilities to simplify and enhance your applications.
No comments yet.