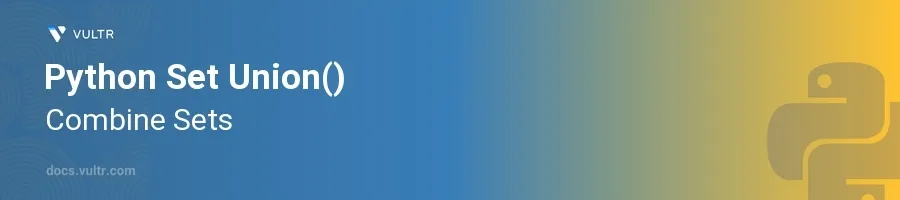
Introduction
The union()
method in Python is a powerful tool for combining sets, allowing for the merger of two or more sets into a single set that contains all elements from the merged sets without duplicates. This is particularly useful in various applications such as data analysis, where combining unique items from multiple sources is frequently required.
In this article, you will learn how to effectively utilize the union()
method in Python to combine sets. Various techniques, including the use of the method with two or more sets, and considerations regarding non-destructive set operations, will be detailed, helping you to manage and combine data collections efficiently.
Basics of Set Union
Combining Two Sets
Define two separate sets with distinct and overlapping elements.
Use the
union()
method to combine these sets into a single set that retains unique elements.pythonset_A = {1, 2, 3, 4} set_B = {3, 4, 5, 6} combined_set = set_A.union(set_B) print(combined_set)
This code results in the combined set
{1, 2, 3, 4, 5, 6}
. Theunion()
merges set_A and set_B, where elements that appear in both sets are included only once.
Union of Multiple Sets
Define more than two sets.
Combine them using the
union()
method by chaining the method or passing all sets as arguments.pythonset_C = {7, 8} set_D = {9, 7} set_E = {10, 11, 8} all_combined = set_A.union(set_B, set_C, set_D, set_E) print(all_combined)
In this example,
all_combined
includes all unique elements from the setsset_A
,set_B
,set_C
,set_D
, andset_E
, resulting in the set{1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11}
.
Advanced Techniques with Set Union
Using Union with Iterable Unpacking
Dynamically unpack iterables containing sets and combine them using the
union()
method.Use the
*
operator for unpacking.pythonlist_of_sets = [set_A, set_B, set_C, set_D, set_E] all_combined_unpack = set().union(*list_of_sets) print(all_combined_unpack)
This approach simplifies the combining of a list or tuple of sets into a single set by unpacking the list
list_of_sets
directly into theunion()
method.
Conditional Union Operations
Filter items during the union operation based on specific conditions.
Use a combination of list comprehensions and the
union()
method.pythoneven_union = set_A.union(x for x in set_B if x % 2 == 0) print(even_union)
This snippet combines
set_A
with only those elements inset_B
that are even, yielding{1, 2, 3, 4, 6}
.
Conclusion
Effectively mastering the union()
method in Python enhances your ability to manage and analyze data across various sets efficiently. By learning to combine sets effectively, preserve data uniqueness, and employ advanced techniques such as iterable unpacking and conditional operations, you optimize data handling and ensure code scalability and readability. Applying these principles allows for streamlined data operations and simplifies complex data manipulation tasks.
No comments yet.