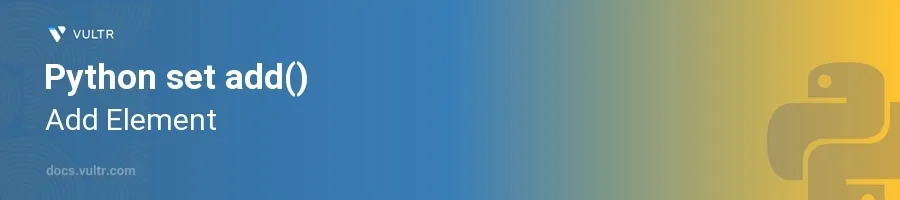
Introduction
The add()
method in Python is a fundamental function of the set data structure, used to append a new element to a set. Since sets are characterized by unique, unordered elements, this method is particularly beneficial when maintaining collections of unique items is necessary, such as when eliminating duplicates dynamically during data processing.
In this article, you will learn how to efficiently use the add()
method in various programming situations. Explore real-world applications of manipulating sets in Python, including working with unique data, managing state, and more complex use cases involving data structures.
Understanding the Basics of Set in Python
Overview of Set Characteristics
Sets in Python provide a way to store unique items. Here are some of their key characteristics:
- Sets are unordered.
- Sets do not allow duplicate elements.
- Sets are mutable, allowing modification after creation.
Using the add() Method
Create a set of numeric elements.
Use the
add()
method to insert a new element into the set.pythonmy_set = {1, 2, 3} my_set.add(4) print(my_set)
This code initializes a set with the elements
{1, 2, 3}
and adds the element4
. Since4
is not previously in the set, it is appended tomy_set
.
Practical Examples of Using add()
Managing Unique Collections
Define a scenario involving a collection of items where duplicates must be avoided.
Utilize the
add()
method to ensure all elements are unique.pythoninventory = set() items_to_add = ['apple', 'banana', 'apple', 'orange'] for item in items_to_add: inventory.add(item) print(inventory)
In this example, attempting to add 'apple' twice does not affect the set, as sets inherently prevent duplicates. This results in a unique collection of items.
Combining Sets with add()
Create two sets representing different groups of elements.
Use the
add()
method in a loop to effectively combine two sets.pythonset_a = {1, 2, 3} set_b = {4, 5, 6} for item in set_b: set_a.add(item) print(set_a)
The loop iteratively adds elements from
set_b
intoset_a
, merging them into a single unique set.
Advanced Usage of add() in Data Processing
Working with Data from Multiple Sources
Collect data from various sources that may include repeated elements.
Apply
add()
to consolidate the elements into a set for unique representation.pythondata_sources = [ [1,2,3], [3,4,5], [6,7,8] ] unique_data = set() for dataset in data_sources: for number in dataset: unique_data.add(number) print(unique_data)
This snippet processes lists from
data_sources
, and each number is added to theunique_data
set, ensuring all numbers are unique despite potential repetition in the input lists.
Conclusion
The add()
method in Python sets is an efficient and straightforward way to manage unique elements within a collection. Whether handling simple sets or managing complex data structures, utilizing add()
enhances the control over data uniqueness and quality. This tutorial explored various applications of the add()
method from basic usage to more intricate scenarios involving data consolidation and management. By mastering the add()
method, make sure data integrity and simplicity in data management are maintained effectively in your Python projects.
No comments yet.