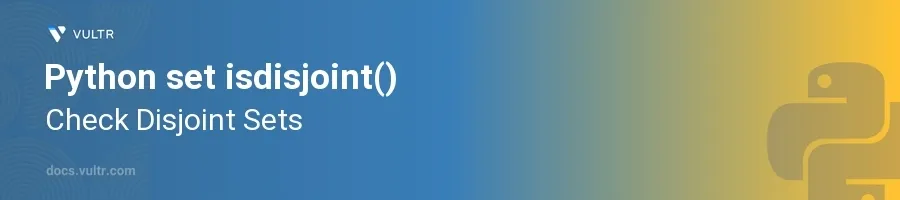
Introduction
The isdisjoint()
method in Python plays a crucial role when working with sets. This method efficiently determines whether two sets have no elements in common, that is, if they are disjoint. It's extremely valuable for tasks such as checking overlapping memberships in data analysis, ensuring uniqueness in collections, or simply comparing sets for mutual exclusiveness.
In this article, you will learn how to harness the isdisjoint()
method across various use cases involving sets. Discover methods for validating the disjoint nature of sets in both basic and advanced contexts, and see how this functionality can be applied in real-world scenarios.
Understanding the isdisjoint() Method
Basic Usage of isdisjoint()
Define two sets.
Use the
isdisjoint()
method to check if these sets are disjoint.pythonset1 = {1, 2, 3} set2 = {4, 5, 6} disjoint_result = set1.isdisjoint(set2) print(disjoint_result)
The sets
set1
andset2
here contain no common elements, soisdisjoint()
returnsTrue
.
Validate Overlapping Sets
Define two sets where some elements overlap.
Apply the
isdisjoint()
method.pythonset1 = {1, 2, 3} set2 = {3, 4, 5} disjoint_result = set1.isdisjoint(set2) print(disjoint_result)
In this case, because both sets share the element
3
,isdisjoint()
returnsFalse
indicating that the sets are not disjoint.
Advanced Use Cases of isdisjoint()
Using isdisjoint() with Multiple Sets
Define multiple sets.
Utilize
isdisjoint()
in a pairwise comparison to check disjointness among all sets.pythonset1 = {1, 2, 3} set2 = {4, 5, 6} set3 = {7, 8, 9} all_disjoint = all(set1.isdisjoint(other_set) for other_set in [set2, set3]) print(all_disjoint)
By employing a combination of
isdisjoint()
and Python'sall()
function with a loop, this code snippet evaluates ifset1
is disjoint with bothset2
andset3
, returningTrue
.
Cross-Referencing Data Sets
Assume sets represent different datasets or categorical groups.
Check for disjointness to confirm exclusive categories.
pythoncustomers_north = {'Alice', 'Bob', 'Clara'} customers_south = {'Xavier', 'Yara', 'Zane'} geographic_disjoint = customers_north.isdisjoint(customers_south) print(geographic_disjoint)
The sets represent customers from northern and southern regions. Since there are no common customers, the sets are disjoint, and the method returns
True
.
Practical Applications in Programming
Ensuring Unique Permissions
Use
isdisjoint()
to ensure that sets of permissions assigned to different user roles do not overlap.Define sets representing permissions of different user roles.
pythonadmin_permissions = {'edit', 'delete', 'create'} viewer_permissions = {'view'} permissions_disjoint = admin_permissions.isdisjoint(viewer_permissions) print(permissions_disjoint)
Here, disjointness checks help maintain clear role-based access control by verifying that viewers do not have editing or creating capabilities.
Data Partitioning in Analysis
Divide data into subsets for analysis without overlap.
Apply
isdisjoint()
to validate the exclusivity of data partitions.pythondata_set_1 = {'data1', 'data2', 'data3'} data_set_2 = {'data4', 'data5'} partition_disjoint = data_set_1.isdisjoint(data_set_2) print(partition_disjoint)
Checking disjointness in this context ensures that data sets do not overlap, which is critical for certain types of statistical analysis or data processing tasks.
Conclusion
The isdisjoint()
method in Python sets is a powerful tool for determining whether two sets are completely separate with no common elements. By integrating this method into your Python toolkit, you can effectively handle situations requiring checks on overlapping or exclusive memberships. Whether you're validating user roles, comparing datasets, or managing any groups of elements, understanding how to use isdisjoint()
boosts your capability to work efficiently and accurately with sets. Implement the strategies shown here to make your programs more robust and reliable.
No comments yet.