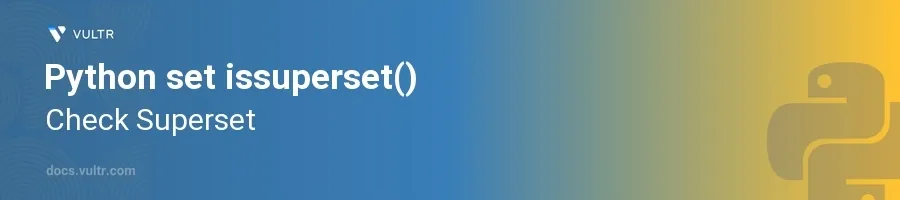
Introduction
The issuperset()
method in Python is a crucial tool for set operations, allowing you to verify whether one set contains all elements of another set. This method is fundamental in mathematics and computing where set theory is used to solve various problems involving collections of items without any particular order.
In this article, you will learn how to effectively use the issuperset()
method. Discover techniques to determine if a set is a superset of another and explore scenarios where variations of data and set sizes influence the outcome of this method.
Understanding issuperset()
issuperset()
can be approached in several ways, and understanding its basic functionality is the first step to mastering its applications. Here’s how you can leverage its capabilities:
Basic Usage of issuperset()
Create two sets, one of which is a potential superset of the other.
Utilize the
issuperset()
method to ascertain if the first set contains all elements of the second set.pythonset_a = {1, 2, 3, 4, 5} set_b = {1, 2, 3} super_check = set_a.issuperset(set_b) print(super_check)
This code establishes
set_a
as a superset ofset_b
because all elements ofset_b
are present inset_a
, thus returningTrue
.
Comparing Different Data Types
Acknowledge that sets can contain various data types.
Test the
issuperset()
method with sets that include mixed data types.pythonset_a = {1, 2, "banana", (3, 4)} set_b = {1, "banana"} result = set_a.issuperset(set_b) print(result)
Here,
set_a
contains integers, a string, and a tuple, whileset_b
comprises an integer and a string. The method returnsTrue
as all elements ofset_b
exist inset_a
.
Practical Applications
Utilizing the issuperset()
method extends beyond simple checks. It can be instrumental in more complex scenarios such as data analysis, permission systems, and inventory controls.
Using issuperset() in Data Analysis
Use
issuperset()
to compare datasets when one should potentially include all elements of another.Employ this method in preprocessing to ensure data consistency.
pythonrequired_fields = {"id", "name", "date"} incoming_data = {"name", "id", "date", "location", "description"} is_consistent = incoming_data.issuperset(required_fields) print(is_consistent)
Ensuring that
incoming_data
contains at least therequired_fields
is crucial for data consistency across systems.
Applying issuperset() in Access Control
Define sets representing user permissions and required permissions for operations.
Determine if a user's permissions set is a superset of the operation's required permissions.
pythonuser_perms = {"edit", "view", "delete"} del_operation_req = {"delete"} can_delete = user_perms.issuperset(del_operation_req) print(can_delete)
Checking if user permissions are sufficient for certain operations can be streamlined with
issuperset()
, enhancing the security and efficiency of the system.
Conclusion
The issuperset()
function in Python offers a straightforward yet powerful way to determine if one set is a superset of another, playing a significant role in applications requiring checks on collections of items. By mastering its use in different contexts and with varying data types, you ensure that your applications handle sets effectively and perform crucial checks in areas like data validation and permission controls. Utilize the issuperset()
method as described in the examples to create robust, efficient programs that utilize set theory optimally.
No comments yet.