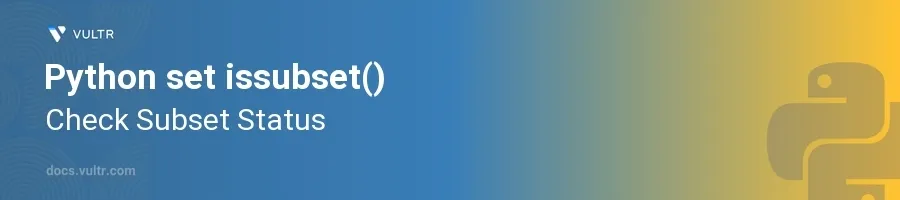
Introduction
Python provides various built-in methods to handle sets efficiently, and one such method is issubset()
. This method is vital when you need to ascertain whether all elements of one set are present in another, effectively checking if one set is a subset of the other. This capability is particularly useful in scenarios involving mathematical sets, permissions, features, or any context where membership needs verification.
In this article, you will learn how to use the issubset()
method with sets in Python. You'll explore different ways to check subset relationships and understand how to apply this method with various data types and conditions. By the end of this article, you'll be comfortable implementing this method to compare sets efficiently in your Python projects.
Exploring the issubset() Method
Basic Usage of issubset()
Start by creating two sets, where one is a probable subset of the other.
Use the
issubset()
method to determine if the first set is a subset of the second.pythonA = {1, 2, 3} B = {1, 2, 3, 4, 5, 6} result = A.issubset(B) print(result)
This code evaluates whether set
A
is a subset of setB
. Since all elements ofA
are indeed elements ofB
,issubset()
returnsTrue
.
Checking Empty Sets
Understand that an empty set is a subset of every set, including another empty set.
Create an empty set and another set and check the subset relationship.
pythonempty_set = set() sample_set = {10, 20, 30} is_subset = empty_set.issubset(sample_set) print(is_subset)
In this example,
empty_set.issubset(sample_set)
returnsTrue
because an empty set is always considered a subset of any set.
Using Superset to Check Subset Condition
Sometimes it's more readable or logical to check if one set is a superset of another.
Instead of using
issubset
, use theissuperset()
method from the perspective of the larger set.pythonprimary_set = {1, 2, 3, 4, 5, 6} secondary_set = {2, 4} result = primary_set.issuperset(secondary_set) print(result)
Here,
primary_set.issuperset(secondary_set)
checks ifprimary_set
contains all elements ofsecondary_set
, returningTrue
when successful.
Using issubset() with Other Iterables
Set operations aren't limited strictly to other sets; they can be conducted with any iterable.
Check if a set is a subset of a list, tuple, or another collection after converting it to a set.
pythonset_A = {3, 4} list_B = [1, 2, 3, 4, 5, 6] result = set_A.issubset(set(list_B)) print(result)
Although
list_B
is not a set, converting it to a set allowsset_A.issubset(set(list_B))
to function correctly, verifying the subset status asTrue
.
Working with Complex Sets
Checking Subset Status in Nested Sets
Nested sets or sets containing tuples can also be part of subset checks.
Test this relationship with composite elements within the sets.
pythoncomplex_A = {(1, 2), (3, 4)} complex_B = {(1, 2), (3, 4), (5, 6)} is_subset = complex_A.issubset(complex_B) print(is_subset)
In the given example,
complex_A
is a subset ofcomplex_B
as all tuple elements incomplex_A
are found incomplex_B
, thus the output isTrue
.
Conclusion
The issubset()
function in Python is a robust tool for checking the subset relationship between sets, enhancing the ability to manage group memberships, permissions, and feature sets efficiently. By understanding and implementing the issubset()
method, you enable more precise control over data groupings and membership verifications in your programming tasks. Utilize these techniques to ensure more structured, logical, and clean code in your Python projects. By mastering these subset checks, you uphold high standards of data integrity and manipulation within your applications.
No comments yet.