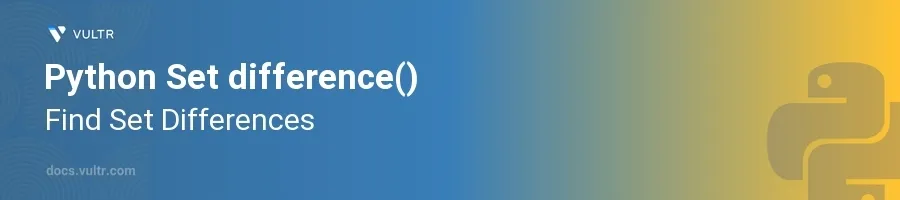
Introduction
In Python, the difference()
method offers a straightforward way to find the differences between two sets, essentially helping to identify elements that are unique to a particular set when compared to another. This method plays a crucial role in data manipulation and analysis, especially when dealing with unique collections of data. Understanding how to use the difference()
method efficiently allows you to handle operations related to set theory with ease.
In this article, you will learn how to utilize the difference()
method to find differences between multiple sets effectively. You will explore several examples that demonstrate the method's utility in handling common scenarios in data processing and set operations.
Understanding the difference() Method
Basic Usage of difference()
Create two sets of elements.
Apply the
difference()
method to identify unique elements in the first set.pythonset1 = {1, 2, 3, 4, 5} set2 = {4, 5, 6, 7} unique_to_set1 = set1.difference(set2) print(unique_to_set1)
This code snippet will display the elements
{1, 2, 3}
. These are the items that are unique toset1
and not found inset2
.
Visualizing Difference Between Multiple Sets
Extend the use of
difference()
to more than two sets.Evaluate the difference successively across multiple sets.
pythonset1 = {1, 2, 3, 4, 5} set2 = {3, 4, 5, 6, 7} set3 = {5, 6, 7, 8, 9} result = set1.difference(set2, set3) print(result)
Here, the output will be
{1, 2}
. The method evaluates the unique elements inset1
that aren't in eitherset2
orset3
.
Practical Applications of difference()
Performing Data Filter Operations
Use
difference()
to filter unwanted data from a dataset.Simulate a scenario where you need to remove blacklisted elements from a set of inputs.
pythonapproved_items = {'apple', 'banana', 'cherry', 'date'} blacklisted_items = {'date', 'fig', 'grape'} safe_items = approved_items.difference(blacklisted_items) print(safe_items)
The output
{apple, banana, cherry}
shows remaining items after removing the blacklisted ones.
Set Difference in Data Analysis
Apply
difference()
to differentiate datasets in analysis.Illustrate with an example of comparing old and updated datasets to find discrepancies.
pythonprevious_data_set = {101, 102, 103, 104} current_data_set = {102, 103, 104, 105} discontinued_items = previous_data_set.difference(current_data_set) print(discontinued_items)
In this example, you identify
{101}
as the discontinued item number, which is no longer present in the current dataset.
Conclusion
The difference()
function in Python is an invaluable tool for managing and analyzing data involving sets. It enables the identification of unique elements, provides a means to carry out comprehensive comparisons, and assists in filtering operations. By mastering this function, you ensure precision and efficiency in data handling tasks that require differentiation between collections. Armed with the knowledge from this tutorial, your journey with Python's set operations will facilitate smoother and more effective programming solutions.
No comments yet.