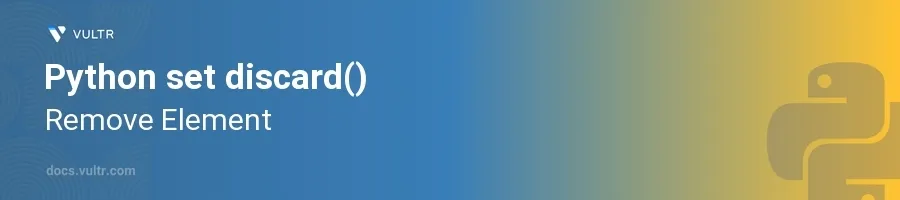
Introduction
The discard()
method in Python is a crucial part of the set methods, enabling the removal of individual elements without causing errors if the element does not exist. This method provides a safe way to delete items from a set, ensuring that the program continues to run smoothly regardless of the element's presence in the set.
In this article, you will learn how to effectively use the discard()
method in various scenarios involving sets. Gain insights into the subtle nuances of this method compared to other removal methods like remove()
, and explore practical examples to deepen your understanding of set manipulation in Python.
Understanding the discard() Method
Basics of discard()
Recognize that the
discard()
method modifies the set in-place by removing the specified element.Note that no error or exception is raised if the element is not found in the set.
pythonmy_set = {1, 2, 3, 4, 5} my_set.discard(3) print(my_set)
This code removes the element
3
frommy_set
. The resulting set will be{1, 2, 4, 5}
.
Comparison with remove()
Understand that
remove()
also deletes an element from the set.Remember that unlike
discard()
,remove()
raises aKeyError
if the element is not present.pythonmy_set = {1, 2, 3, 4, 5} try: my_set.remove(6) except KeyError: print("Element not found")
In this code, attempting to remove
6
which isn't inmy_set
causes aKeyError
.
When to Use discard()
Use
discard()
when uncertain if the element is in the set and want to avoid an exception.Prefer it for larger scripts or applications where stability and error handling are concerns.
pythondata_set = set('hello world') characters_to_remove = 'hl' for char in characters_to_remove: data_set.discard(char) print(data_set)
This process removes characters 'h' and 'l' from the set without the risk of a
KeyError
if they are not found.
Practical Applications of discard()
Cleaning Up Data Sets
Consider using
discard()
for preprocessing tasks in data analysis, such as filtering unwanted data points.Implement it when the presence of specific outlier elements is uncertain.
pythonage_set = {22, 25, 29, 34, 40, 45, 50, 55, 120} age_set.discard(120) # Assuming 120 is an outlier print(age_set)
Removing the outlier
120
simplifies the dataset for further analysis.
Interactive Programs
Utilize
discard()
in interactive environments where users can dynamically alter the data set.Handle scenarios where users may try to remove non-existing elements without causing interruptions.
pythonuser_set = {1, 2, 3, 4, 5} user_input = int(input("Enter a number to discard: ")) user_set.discard(user_input) print(f"Updated set: {user_set}")
This interactive console program lets the user attempt to discard an element from
user_set
, safely handling non-existent entries.
Conclusion
Mastering the discard()
method in Python sets is vital for developing robust programs that handle data element manipulation safely and effectively. By employing discard()
in appropriate situations, you avoid unnecessary errors and ensure smoother program execution. Whether cleaning datasets, managing user input, or ensuring stability in critical systems, discard()
proves to be invaluable. Apply the explained strategies and examples to make the most out of this method in your own Python projects, enhancing both code quality and resilience.
No comments yet.