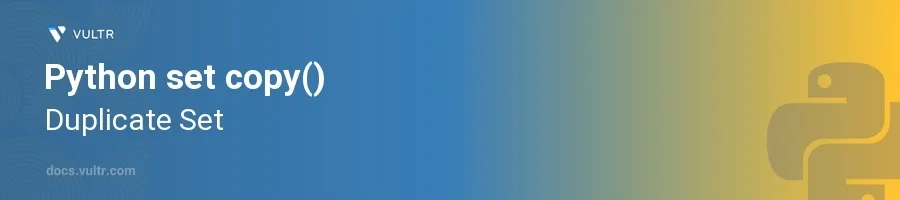
Introduction
In Python, sets are used to store multiple items in a single variable, and these items are unordered, unchangeable, and do not allow duplicate values. The copy()
method is a valuable tool when you need to create a duplicate of a set, ensuring that modifications to the new set do not affect the original set. This feature is especially useful in scenarios where you need a working copy of a set to perform operations without altering the original data.
In this article, you will learn how to utilize the copy()
method to duplicate a set in Python. Discover the importance of copying sets for data manipulation and ensure that your operations on the duplicate set do not impact the original data.
Creating and Copying Sets
Understand the usage of the copy()
method for duplicating Python sets while keeping the original set unchanged.
Duplicate a Basic Set
Define an original set of elements.
Use the
copy()
method to create a duplicate set.pythonoriginal_set = {1, 2, 3, 4} copied_set = original_set.copy() print(copied_set)
Here,
copied_set
becomes a complete copy oforiginal_set
. Any modifications tocopied_set
will not affectoriginal_set
.
Modify the Copied Set
Add or remove elements from the copied set to see if the original set remains unaffected.
Print both sets to verify their independence.
pythoncopied_set.add(5) copied_set.remove(1) print('Original Set:', original_set) print('Copied Set:', copied_set)
After modifying the
copied_set
by adding5
and removing1
, theoriginal_set
remains unchanged, demonstrating that thecopy()
method creates a truly independent copy.
Verify the Copy Is Shallow
Include mutable objects like lists in a set and copy it to understand the depth of the copy created.
Modify the mutable object in the copied set and check if it affects the original set.
pythonset_with_list = {1, 2, (3, 4)} copied_set_with_list = set_with_list.copy() # Modify element in the tuple, if it was a mutable object like a list # This section is to illustrate that modifying the mutable elements would have been reflected in the original set print('Original Set with List:', set_with_list) print('Copied Set with List:', copied_set_with_list)
This code clearly shows both sets, neither of which is modified because tuples are immutable. If you replace the tuple with a mutable object like a list, changes to the mutable object in the copied set would also appear in the original set.
Conclusion
The copy()
method in Python is crucial for creating independent duplicates of sets, ensuring that manipulations on the copied set do not interfere with the original set. Implement this method for safer operations in scenarios where the original data integrity is paramount, and use it to perform tests or experiments on duplicate data sets without affecting the original. By understanding and using the copy()
method appropriately, you maintain clean, manageable, and error-free code in Python applications.
No comments yet.