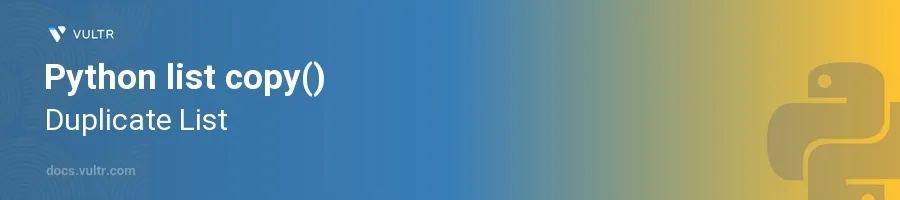
Introduction
The copy()
method in Python is a crucial tool for duplicating lists. Often, when working with lists, there's a need to create a new list that mimics the original without altering it. This requirement becomes significant, especially when lists contain mutable objects, and modifications to the original list must be avoided without unintended side effects.
In this article, you will learn how to use the copy()
method effectively to duplicate lists in Python. Explore the nuances of shallow copying compared to other copying techniques and understand its practical applications with clear examples.
Using List copy() Method
Duplicate a Simple List
Define a list of simple elements like integers or strings.
Use the
copy()
method to make a duplicate of the list.pythonoriginal_list = [1, 2, 3, 4] duplicated_list = original_list.copy() print(duplicated_list)
This code duplicates
original_list
using thecopy()
method, and changes toduplicated_list
will not affectoriginal_list
.
Impact on Mutable Objects
Understand that
copy()
creates a shallow copy.Create a list containing mutable objects like lists.
Duplicate the list and modify the nested list to see the effect.
pythonnested_list = [[1, 2], [3, 4]] copied_list = nested_list.copy() copied_list[0][0] = 9 print("Original List:", nested_list) print("Copied List:", copied_list)
Here, modifying the sublist in
copied_list
also affectsnested_list
becausecopy()
creates a shallow copy where nested objects are referenced.
Using copy() in List Comprehensions
Combine list comprehensions with
copy()
for efficient duplications.Use a simple condition or transformation during copying.
pythonlist_with_comprehension = [x*2 for x in original_list.copy()] print(list_with_comprehension)
This snippet duplicates
original_list
while doubling each element during the copying process, illustrating how to combine list comprehension with copying.
Conclusion
The copy()
method is essential for duplicating lists in Python, especially when handling complex data structures containing mutable types. Utilize this method to ensure modifications to new lists do not impact the original data. By mastering copy()
, you ensure data integrity in your applications while effectively managing list duplications. Whether duplicating simple lists or those containing nested mutable items, copy()
provides a straightforward and efficient solution.
No comments yet.