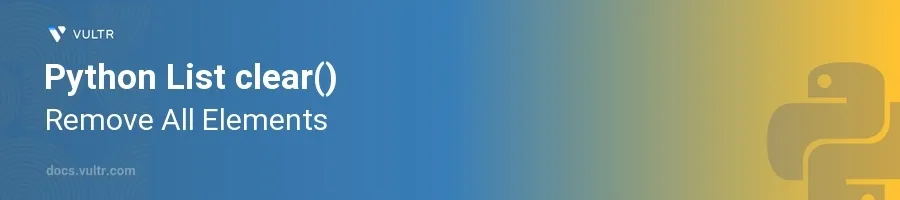
Introduction
The clear()
method in Python is a straightforward and efficient way to remove all items from a list. This method is useful in scenarios where you need to reuse an already defined list without holding onto the old data, making it optimal for tasks that require frequent reset of list contents without creating new list objects.
In this article, you will learn how to effectively use the clear()
method to manage list data in Python. Understand how this method operates on various types of lists and how it impacts the list and its references.
Understanding clear()
Basic Usage of clear()
Initialize a list with multiple elements.
Apply the
clear()
method to remove all elements.pythonfruits = ['apple', 'banana', 'cherry', 'date'] fruits.clear() print(fruits)
This code will output an empty list
[]
asclear()
removes all items from thefruits
list.
Impact on References
Create a list and assign it to another variable.
Use the
clear()
method on the original list.Check the content of the second variable.
pythonoriginal_list = [1, 2, 3, 4] reference_list = original_list original_list.clear() print(reference_list)
The output will be an empty list
[]
. Bothoriginal_list
andreference_list
refer to the same list object; thus, clearing one affects all references.
Use Cases for clear()
Reusing List Containers
Consider scenarios where memory efficiency is critical.
Use
clear()
instead of reassigning a new list to the same variable to manage memory more effectively.Reusing the same list after clearing its contents can often be more memory-efficient than creating a new list, especially in a loop or when handling large data sets.
Resetting Data
Utilize
clear()
in applications like game developments or simulations where reset of state is frequently needed.Clear lists that store temporary data between sessions or cycles without creating new lists.
This practice enhances performance by reducing the overhead associated with memory allocation and garbage collection.
Conclusion
The clear()
method in Python lists is an essential function for efficiently managing list contents, particularly useful in applications requiring frequent resets or memory-efficient data handling. By utilizing clear()
, you maintain cleaner code, enhance performance through optimal memory usage, and ensure that changes to a list affect all references consistently. Implement this methodology in your Python projects to streamline list management and avoid unnecessary memory clutter.
No comments yet.