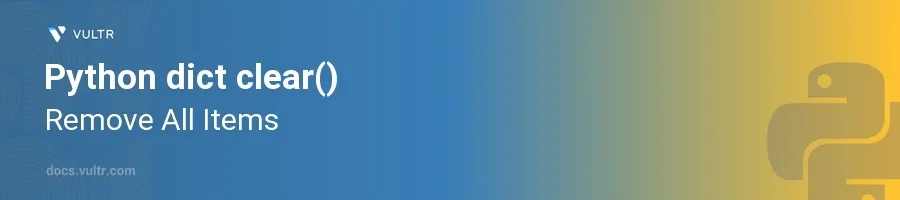
Introduction
The clear()
method in Python is a crucial tool for dictionary management, allowing users to remove all items from a dictionary quickly and efficiently. This method, part of the Python dict clear functionality, proves invaluable when you need to reset a dictionary during runtime, such as clearing caches or resetting data states in an application.
In this article, you will learn how to use the clear()
method effectively. Explore practical use cases where resetting a dictionary is necessary and how incorporating the python dict.clear()
method helps with clear a dictionary in python operations, streamlining code and enhancing performance in applications.
How to Clear a Dictionary in Python Using dict clear()
Clear a Dictionary Completely
Define a dictionary filled with several key-value pairs.
Apply the
clear()
method to empty the dictionary.pythonmy_dict = {'name': 'Alice', 'age': 25, 'location': 'New York'} my_dict.clear() print(my_dict)
After executing
my_dict.clear()
, the dictionarymy_dict
becomes empty, resulting in an output of{}
when printed.
Use clear() in a Function
Setup a function that manipulates a dictionary.
Clear the dictionary before exiting the function to ensure no residual data persists.
pythondef process_data(): data = {'key1': 100, 'key2': 200, 'key3': 300} # Perform some operations (example simulated by a print statement) print("Processing:", data) data.clear() # Dictionary is cleared before function exits print("Data after clearing:", data) process_data()
This code block defines a function
process_data()
which initially processes some data, then usesdata.clear()
to erase all contents of the dictionary, ensuring that no data leaks outside the scope of the function.
Prevent Memory Leaks in Loops
Use the
clear()
method within a loop to maintain minimal memory footprint when repeatedly modifying dictionaries.pythonfor _ in range(3): temporary_data = {'id': _, 'value': _ * 10} print("Temporary data:", temporary_data) temporary_data.clear()
By incorporating
temporary_data.clear()
within the loop, each dictionary instance gets cleared at the end of its cycle, preventing memory accumulation or overhead.
Conclusion
Utilizing the clear()
method in Python dictionaries provides you with an effective way to manage and reset dictionary contents efficiently. Whether you're dealing with function scopes, loops, or large-scale data manipulations, incorporating clear()
enhances both the safety and performance of your applications. Emphasize clean and efficient code by harnessing the power of dictionary management with the clear()
method.
No comments yet.