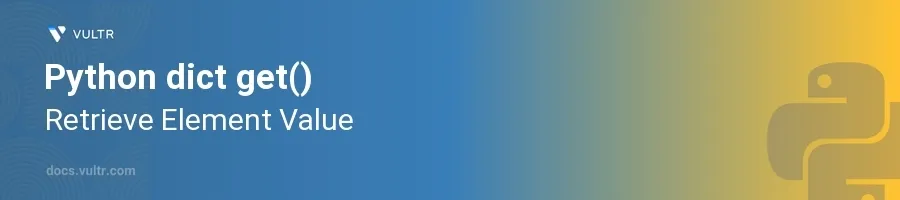
Introduction
The get()
method in Python's dictionary is a fundamental tool for retrieving values associated with specified keys. This method provides a safe way to access dictionary items without risking a KeyError, which can occur if you try to access a key that does not exist in the dictionary using the standard square bracket notation.
In this article, you will learn how to proficiently use the dict.get()
method to access dictionary values in Python. Expand your understanding of this method by exploring examples that demonstrate how to use it effectively in different scenarios, including handling missing keys gracefully.
Utilizing dict.get()
Retrieve a Value with a Default Return
Acces a dictionary by specifying the key.
Assign a default value that
get()
should return if the key does not exist.pythonplayer_scores = {'Alice': 88, 'Bob': 95} score = player_scores.get('Carol', 0) print(score)
In this code,
player_scores.get('Carol', 0)
returns0
because 'Carol' is not a key inplayer_scores
. The default value0
is used.
Handling None Default Values
Use
get()
to access a key without providing a default value.Observe that
None
is returned if the key doesn't exist.pythonplayer_scores = {'Alice': 88, 'Bob': 95} score = player_scores.get('Carol') print(score)
If a second parameter (default value) is not provided,
get()
returnsNone
when the key is absent, as seen for 'Carol'.
Practical Applications of dict.get()
Data Filtering Based on Presence of Keys
Assume a scenario where filtering data requires checking if certain keys exist.
Use
get()
to simplify conditional checks.pythondata = {'id': 102, 'name': 'John Doe', 'email': 'john@example.com'} if data.get('email'): print('Email exists:', data['email']) else: print('No email provided.')
This script checks if 'email' exists without causing an error if it does not. It directly uses
get()
to safely attempt to retrieve the 'email' key.
Combining dict.get() in Dictionary Comprehensions
Create a more dynamic retrieval of values using dictionary comprehensions.
Integrate
get()
within the comprehension for safer data extraction.pythonusers = [ {'name': 'Alice', 'role': 'admin'}, {'name': 'Bob', 'role': 'editor'}, {'name': 'Charlie'} ] roles = {user.get('name'): user.get('role', 'guest') for user in users} print(roles)
This builds a new dictionary
roles
where each 'name' from theusers
list acts as a key. If a user lacks a 'role', it assigns 'guest' by default. This use ofget()
avoids errors and provides fallback values efficiently.
Conclusion
The get()
function in the Python dictionary allows for safe and flexible retrieval of values, making it an indispensable tool for handling data that might not always be complete or uniform. It offers a reliable alternative to direct key access, enhancing code robustness and readability. Apply the get()
method in various programming contexts, especially where the presence of every key is not guaranteed, ensuring your applications handle missing data gracefully and effectively.
No comments yet.