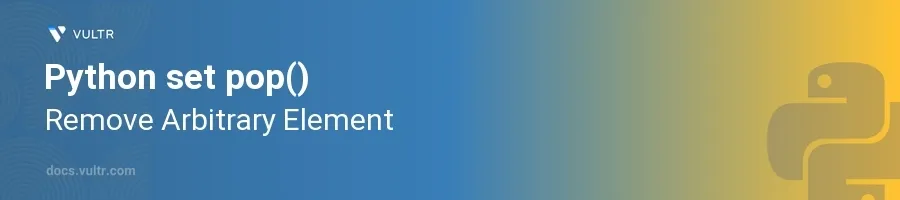
Introduction
The pop()
method in Python's set
data structure is a technique for removing an arbitrary element. This function is essential for occasions when the specific order of elements does not matter, but one random element needs to be removed effectively, such as while implementing certain algorithms or managing state without a predefined order.
In this article, you will learn how to leverage the pop()
method in various scenarios associated with set manipulation. Explore the foundational usage of pop()
and see practical examples of how to incorporate it into Python programs dealing with sets.
Basic Usage of pop()
Remove a Random Element
Create a set with various elements.
Use the
pop()
method to remove a random element and print the modified set.pythonmy_set = {1, 2, 3, 4, 5} popped_element = my_set.pop() print("Popped Element:", popped_element) print("Updated Set:", my_set)
In this snippet,
pop()
removes a random element frommy_set
. The exact item removed depends on the internal ordering of the set, which is unpredictable. The method returns the removed element and the set updates to reflect this change.
Handling an Empty Set
Acknowledge that popping from an empty set raises an error.
Use a try-except structure to handle this potential error gracefully.
pythonempty_set = set() try: empty_set.pop() except KeyError: print("Attempted to pop from an empty set.")
This code snippet tries to
pop()
fromempty_set
, catching theKeyError
that Python raises if the set is empty. This approach prevents the program from crashing and allows it to handle the error appropriately.
Advanced Usage of pop()
Using pop() in Loop Conditions
Utilize
pop()
within a loop to process elements until the set is empty.Combine
pop()
with other operations like conditional checks or accumulating results.pythonnum_set = {10, 20, 30, 40, 50} sum_result = 0 while num_set: element = num_set.pop() if element % 20 == 0: sum_result += element print("Sum of multiples of 20:", sum_result)
This example demonstrates the use of
pop()
in a loop to process elements ofnum_set
until it's depleted. It selectively adds elements that are multiples of 20 tosum_result
. This methodology is useful for reducing a set based on specific criteria.
Integrating pop() with Other Data Structures
Mix
pop()
with structures like lists or dictionaries for complex data manipulations.Use the popped element from a set to perform operations involving other data types.
pythonchar_set = {'a', 'b', 'c'} index_dict = {'a': 1, 'b': 2, 'c': 3} indices = [] while char_set: char = char_set.pop() if char in index_dict: indices.append(index_dict[char]) print("Indices collected:", indices)
This code removes elements from
char_set
and gathers corresponding indices fromindex_dict
into the listindices
. Such integration is effective for synchronizing actions across different types of collections based on the dynamics of sets.
Conclusion
The pop()
method of Python sets is highly valuable when you need to remove elements unpredictably, supporting a variety of practical and algorithmic applications. By mastering the usage scenarios and combining them with other Python functionalities, optimize the management of unordered collections. Always be cautious of empty sets to avoid errors and adapt pop()
effectively in broader contexts, demonstrating its versatility in Python programming.
No comments yet.