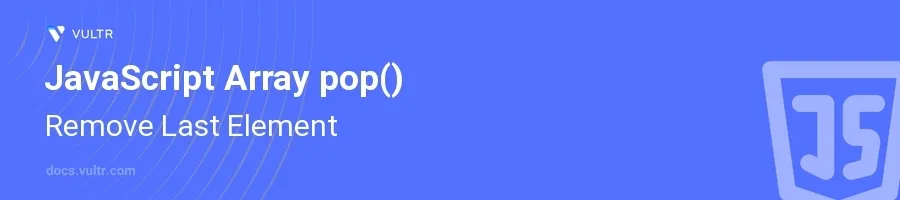
Introduction
The pop()
method is a fundamental array operation in JavaScript, designed to remove the last element from an array. This method not only alters the original array by removing its last element but also returns that element, which can be useful for many programming tasks involving data structures.
In this article, you will learn how to use the pop()
method to manage arrays effectively. Explore various scenarios where pop()
proves beneficial, from basic array manipulation to more complex data handling cases.
Basic Usage of pop()
Removing the Last Element
Initialize an array with multiple elements.
Apply the
pop()
method to remove the last element.javascriptlet fruits = ['Apple', 'Banana', 'Cherry']; let removedElement = fruits.pop(); console.log(fruits); console.log(removedElement);
This code removes 'Cherry' from the
fruits
array. Afterpop()
executes,fruits
contains only ['Apple', 'Banana'], and 'Cherry' is stored inremovedElement
.
Checking the Modified Array
Print the altered array to confirm the change.
Display the removed element.
Following the previous example, the console output will show the updated array and the element that was removed.
Advanced Usage of pop()
Manipulating Elements in Loops
Use a loop to remove elements until the array is empty.
Exhibit each step to understand how
pop()
interacts with array operations dynamically.javascriptlet numbers = [1, 2, 3, 4, 5]; while (numbers.length > 0) { let removed = numbers.pop(); console.log('Removed:', removed); console.log('Remaining Array:', numbers); }
In this scenario,
pop()
is called repeatedly within a while loop, which runs until the array is empty. Each iteration removes the last element and prints its value along with the remaining array.
Combining pop() with Conditionals
Utilize
pop()
in conjunction with conditional statements to perform selective removals based on specific criteria.Keep manipulating the array until a particular condition is met.
javascriptlet scores = [75, 80, 95, 60, 88]; while (scores.length > 0 && scores[scores.length - 1] >= 80) { console.log('Removing high score:', scores.pop()); } console.log('Final scores:', scores);
This snippet continuously removes elements from the
scores
array as long as the last element of the array is 80 or more. It efficiently usespop()
to both alter the array and supply the conditional loop with the required checks.
Conclusion
The pop()
method in JavaScript is a simple yet powerful array manipulation tool. Its function to remove the last element of an array is crucial for operations where element order and array size are significant. With examples ranging from basic to more advanced, it's clear that pop()
offers a straightforward approach to modifying arrays. Implement these techniques in your scripting to keep data structures dynamic and responsive to runtime conditions.
No comments yet.