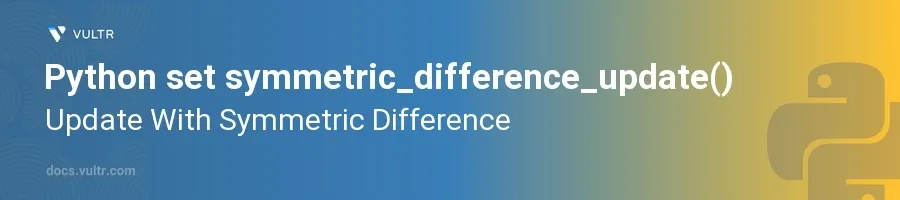
Introduction
The symmetric_difference_update()
method in Python is an integral part of the set operations that allows for in-place modification of a set by keeping only the elements found in either of the sets, but not in both. This method updates the set on which it's called, making it an efficient choice for scenarios where you need to dynamically alter the contents of sets during program execution.
In this article, you will learn how to harness the symmetric_difference_update()
method effectively. You will explore practical examples that demonstrate how to use this method to manipulate and update sets directly, adapting to requirements that involve unique element comparison and retrieval in different sets.
Understanding symmetric_difference_update()
The symmetric_difference_update()
method operates by modifying the set it is called on. This alteration involves retaining only the elements that are unique to each set involved in the operation, effectively removing those that appear in both sets.
Example 1: Basic Usage
Initialize two sets with some common and some unique elements.
Apply
symmetric_difference_update()
on one of the sets.pythonset1 = {1, 2, 3, 4} set2 = {3, 4, 5, 6} set1.symmetric_difference_update(set2) print(set1)
Here,
set1
starts with elements{1, 2, 3, 4}
, andset2
has{3, 4, 5, 6}
. After applyingsymmetric_difference_update()
,set1
is updated to{1, 2, 5, 6}
, which are the elements unique to each set when the method was called.
Example 2: Reflecting Real-world Scenarios
Consider a scenario where two groups of workshop participants are listed, and you need to find out those who are only attending either one but not both workshops.
Use
symmetric_difference_update()
to update the list of attendees for reassignment or notifications.pythonworkshop_A = {'Alice', 'Bob', 'Charlie', 'Diana'} workshop_B = {'Charlie', 'Diana', 'Ethan', 'Fiona'} workshop_A.symmetric_difference_update(workshop_B) print(workshop_A)
This operation results in
workshop_A
being updated to{'Alice', 'Bob', 'Ethan', 'Fiona'}
, representing participants exclusive to each workshop.
Advanced Usage of symmetric_difference_update()
The flexibility of symmetric_difference_update()
allows for its use in more complex data management tasks, such as updating datasets in response to real-time changes or processing user inputs that require set operations.
Streamlining Data Comparison
- Use
symmetric_difference_update()
to handle dynamic datasets where entries might be added or removed frequently. - Continuously update one set based on changes reflected in another set, ensuring that only unique entries remain.
Managing User Input
Collect user inputs where you expect duplicates or overlapping data.
Use
symmetric_difference_update()
to refine these inputs, removing common entries and retaining unique data points.pythonuser_set = {'apple', 'banana', 'cherry'} input_set = {'banana', 'cherry', 'date', 'elderberry'} user_set.symmetric_difference_update(input_set) print(user_set)
After this code executes,
user_set
will contain{'apple', 'date', 'elderberry'}
, removing common fruits and adding unique ones.
Conclusion
The symmetric_difference_update()
method in Python enriches set manipulation by providing in-place updates that help maintain only unique elements from involved sets. This feature is crucial in scenarios requiring efficient data processing and manipulation, such as real-time data comparison and user interaction handling. Master this method to keep your data handling capabilities robust, efficient, and adaptive to changes, ensuring you manage sets effectively in your Python projects.
No comments yet.