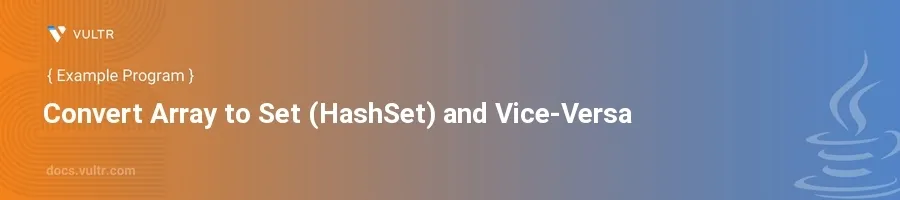
Introduction
Java provides various ways to convert data between common data structures such as arrays and collections like sets. This is essential for situations where different data structures are needed at different stages of application logic—for example, needing the unique elements of an array or wanting faster lookups that a set provides. Converting an array to a set eliminates duplicates because a set stores only unique elements. Conversely, converting a set to an array could be necessary for operations that are possible only on arrays or for API compatibility.
In this article, you will learn how to convert an array into a HashSet
, and then back from a HashSet
to an array. Discover how using the standard Java Collections Framework simplifies these transformations while you gain insights into the performance and usage considerations.
Converting Array to HashSet
Converting an array to a HashSet
in Java includes a straightforward process using the Arrays
and Collections
utilities. This method ensures all duplicate elements are automatically removed.
Step-by-step Conversion
Start by declaring and initializing an array.
Convert this array into a
HashSet
.javaString[] fruitArray = {"apple", "orange", "banana", "apple", "pear"}; HashSet<String> fruitSet = new HashSet<>(Arrays.asList(fruitArray));
This code snippet starts by creating an array of fruits where "apple" appears twice. By passing it into the constructor of
HashSet
throughArrays.asList()
, you create a newHashSet
that contains only unique fruits.
Verification of the Result
Confirm the contents of the
HashSet
to ensure there are no duplicates.javaSystem.out.println(fruitSet);
Executing this line prints the set on the console, which should show each fruit only once, confirming that duplicates like "apple" are removed.
Converting HashSet to Array
After working with sets, you might need to convert a HashSet
back to an array, whether for method compatibility or other operations that require array data structures.
Basic Array Conversion
Declare and initialize a
HashSet
.Convert this
HashSet
back into an array.javaHashSet<String> fruitSet = new HashSet<>(Arrays.asList("apple", "orange", "banana", "pear")); String[] fruitArray_back = fruitSet.toArray(new String[0]); // Zero-length array is fine
Initially, a
HashSet
is created from a list of fruits. ThetoArray(new String[0])
call converts the set back into an array. The zero-length array argument is a type indicator and doesn't need to specify the size because the set defines the resulting array's size.
Verify the Array Output
Print the array to check the results.
javaSystem.out.println(Arrays.toString(fruitArray_back));
This command prints the array, showing it has maintained the unique set elements and converted them back into an array format.
Considerations for Performance and Usage
- Using
HashSet
to remove duplicates from an array is efficient and concise. - Converting between arrays and sets is fast, but it is important to remember that order is not guaranteed in a
HashSet
. - Set operations typically offer faster lookups than arrays, particularly beneficial for large datasets.
Conclusion
Converting between arrays and HashSet
in Java is a useful skill that helps manipulate data structures efficiently according to the needs of your program. This article demonstrated simple methods to convert an array to a HashSet
and back to an array using practical Java code snippets. Implementing these conversions helps manage data effectively, especially when dealing with issues like duplicate data removal or when performing operations that require specific data structures. Mastery of these techniques ensures flexibility and efficiency in your Java applications.
No comments yet.