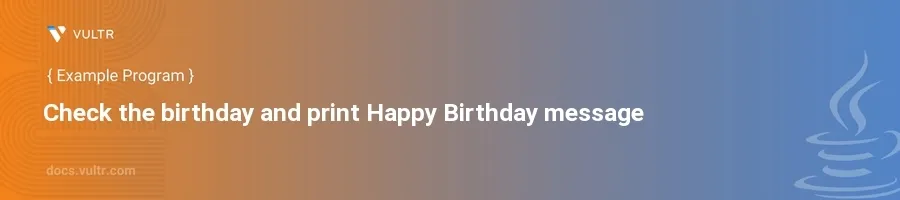
Introduction
Java, a robust and popular programming language, is frequently utilized for building versatile and interactive applications, including those handling date and time operations. One common application is creating programs to check if it’s someone’s birthday and then display a celebratory message. This task not only reinforces basic Java concepts but is also a practical demonstration of date manipulation and condition checks.
In this article, you will learn how to create a Java program that checks if the current date is a specified birthday and prints a "Happy Birthday" message. Walk through detailed examples that illustrate how to handle date operations, compare dates, and implement these in a simple Java application.
Setting Up the Date Comparison
Import Necessary Classes
Import
java.time.LocalDate
andjava.time.Month
to handle date operations in Java.javaimport java.time.LocalDate; import java.time.Month;
The
LocalDate
class represents a date without time and timezone in the ISO-8601 calendar system. TheMonth
enum is used to represent the month-of-year field with ease.
Define the Birthday and Current Date
Create a
LocalDate
object for the birthday.Obtain the current date using
LocalDate.now()
.javaLocalDate birthday = LocalDate.of(1992, Month.MAY, 24); LocalDate currentDate = LocalDate.now();
This code sets up a specific birthday, here, May 24, 1992. It also fetches the current date from the system.
Check if it is the Birthday
Compare Month and Day
Compare the current date's month and day with the birthday month and day.
Print "Happy Birthday" if they match, and "It's not your birthday yet." otherwise.
javaif (currentDate.getMonth() == birthday.getMonth() && currentDate.getDayOfMonth() == birthday.getDayOfMonth()) { System.out.println("Happy Birthday!"); } else { System.out.println("It's not your birthday yet."); }
In this snippet, the program checks if the month and day of the
currentDate
match those of thebirthday
. The use of logical AND ensures both conditions must be true to enter the if block.
Running the Full Program
Complete Java Program
Combine all the steps above into a single Java program to execute.
javaimport java.time.LocalDate; import java.time.Month; public class BirthdayChecker { public static void main(String[] args) { LocalDate birthday = LocalDate.of(1992, Month.MAY, 24); LocalDate currentDate = LocalDate.now(); if (currentDate.getMonth() == birthday.getMonth() && currentDate.getDayOfMonth() == birthday.getDayOfMonth()) { System.out.println("Happy Birthday!"); } else { System.out.println("It's not your birthday yet."); } } }
Run this Java program to check the birthday matching logic. It will print "Happy Birthday!" if today is May 24, and "It's not your birthday yet." otherwise.
Conclusion
The ability to work with dates in Java opens the door to many practical applications, such as creating a program that notifies when it’s someone’s birthday. By constructing this simple birthday checker program, you leverage basic concepts of Java’s date APIs, particularly LocalDate
and condition checks. Apply this knowledge to build more complex date-related operations and reminders in your future Java projects. This straightforward approach not only confirms your understanding of Java dates but also enhances your skill in implementing condition-based messages in your applications.
No comments yet.