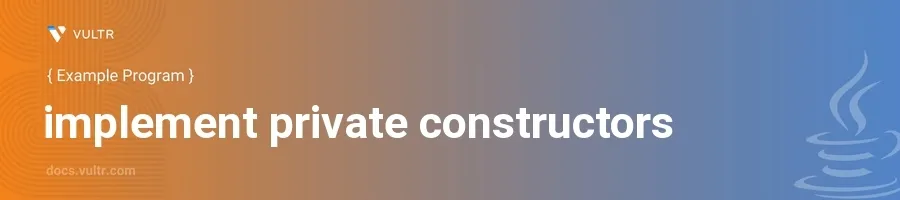
Introduction
Java programming allows the use of private constructors to restrict the instantiation of classes from outside code, ensuring more control over how objects are created. Typically, this feature is utilized in patterns like Singleton, where it's crucial to manage the number of object instances or in utility classes that shouldn't be instantiated at all.
In this article, you will learn how to use private constructors in Java effectively through practical examples. Explore how this can be useful in creating Singleton classes and utility classes to maintain better control and integrity of your application's architecture.
Implementing Private Constructors in Singleton Pattern
The Concept of Singleton Pattern
- Understand the Singleton pattern: It ensures that a class has only one instance and provides a global point of access to it.
- Recognize the need for a private constructor: It prevents the creation of a class instance from any other class.
Example: Creating a Singleton Class
Create a class with a private constructor.
Utilize a static method that provides access to the instance.
javapublic class Singleton { private static Singleton instance; private Singleton() {} public static Singleton getInstance() { if (instance == null) { instance = new Singleton(); } return instance; } }
In this code block, the
Singleton
class uses a private constructor to restrict instantiation. ThegetInstance()
method checks if an instance ofSingleton
already exists; if not, it creates one. This ensures that there is always only one instance ofSingleton
.
Benefits of Using Singleton Pattern
- Ensures that a resource-consuming object isn't created more than once.
- Provides a controlled access point to a shared resource throughout an application, such as a database connection or a configuration manager.
Utilizing Private Constructors in Utility Classes
Understanding Utility Classes
- Recognize utility classes: These are classes typically filled with static methods and constants. They are not meant to be instantiated.
- Know why a private constructor is useful: It prevents the instantiation of utility classes, avoiding improper usage.
Example: Creating a Utility Class
Design a utility class with a private constructor.
Add static methods that do not rely on object state.
javapublic class Utils { // Prevent instantiation private Utils() {} public static int add(int a, int b) { return a + b; } }
Here, the
Utils
class has a private constructor to prevent it from being instantiated. The static methodadd()
provides functionality without needing an instance ofUtils
. This design ensures thatUtils
is used as intended – as a provider of static functionalities.
Usage Scenarios for Utility Classes
- Use for grouping related static operations like mathematical operations, string processing, or file manipulations.
- Employ in cases where maintaining the state is unnecessary or could be harmful.
Conclusion
Using private constructors in Java is a potent technique for enhancing control over how classes are used within software architectures. By implementing private constructors, you promote the intentional use of classes either by restricting their instantiation as in Singleton patterns, or by preventing instantiation altogether as with utility classes. These practices lead to a more robust, maintainable, and error-resistant codebase. Embrace these strategies to capitalize on Java's capabilities, ensuring your programs are efficient and logically organized.
No comments yet.