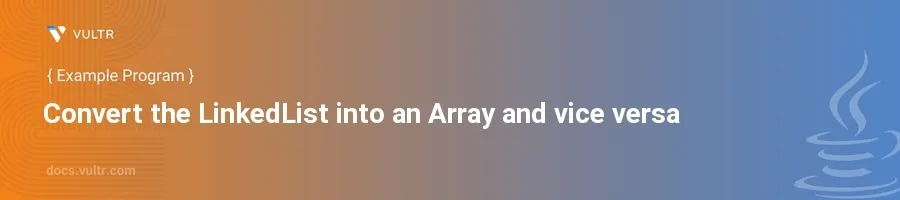
Introduction
Dealing with collections is a fundamental aspect of programming in Java, where LinkedList
and arrays are two of the most utilized data structures. While both have their unique applications, sometimes you need to convert between these two types depending on the requirements of your project or the specific efficiencies they offer. Converting a LinkedList
to an array could be useful for functionalities that require quick random access, whereas transforming an array to a LinkedList
is beneficial for dynamic data manipulation.
In this article, you will learn how to convert a LinkedList
into an array and vice versa using Java. Explore practical examples that not only make these conversions but also help you understand when and why to use each approach effectively in real-world applications.
Convert LinkedList to Array
Converting a LinkedList
to an array in Java is straightforward, thanks to the built-in methods provided by the Java Collections Framework.
Example: LinkedList to Array
Initialize a
LinkedList
.Convert the
LinkedList
to an Array using thetoArray()
method.javaimport java.util.LinkedList; import java.util.Arrays; public class Main { public static void main(String[] args) { LinkedList<String> linkedList = new LinkedList<>(); linkedList.add("Apple"); linkedList.add("Banana"); linkedList.add("Cherry"); String[] array = new String[linkedList.size()]; linkedList.toArray(array); System.out.println("Array: " + Arrays.toString(array)); } }
Here, a
LinkedList
of strings is converted into an array of strings. ThetoArray()
method populates the passed array with the elements of theLinkedList
. The size of the array is set to match the size of theLinkedList
to accommodate all its elements.
Considerations When Converting to Array
- Ensure the array is appropriately sized to hold all elements of the
LinkedList
. - The type of the array should match the type of elements in the
LinkedList
to preventClassCastException
.
Convert Array to LinkedList
The conversion of an array to a LinkedList
involves a couple of extra steps but is equally straightforward with the help of Java's Arrays
class.
Example: Array to LinkedList
Start with an array of elements.
Convert the array to a
LinkedList
usingArrays.asList()
and theLinkedList
constructor.javaimport java.util.LinkedList; import java.util.Arrays; public class Main { public static void main(String[] args) { String[] array = {"Apple", "Banana", "Cherry"}; LinkedList<String> linkedList = new LinkedList<>(Arrays.asList(array)); System.out.println("LinkedList: " + linkedList); } }
In this example,
Arrays.asList()
creates a fixed-size list backed by the specified array, and theLinkedList
constructor then takes this list to initialize a newLinkedList
. The resultingLinkedList
contains all elements of the original array.
Tips for Array to LinkedList Conversion
- Understand that changes to elements in the
List
returned byArrays.asList()
reflect in the original array. - Use this method for collections that need dynamic operations post-conversion, such as element insertion and deletion.
Conclusion
Converting between LinkedList
and arrays in Java is a common task and understanding how to make these conversions efficiently allows for more flexible code. Keep in mind the specific methods available in Java's Collections Framework that aid in these transformations. Whether you need the indexed access provided by arrays or the dynamic nature of LinkedLists
, switching between these data structures efficiently enhances the versatility and performance of your Java applications. By mastering these conversions, you make sure your Java toolkit is well-rounded and prepared for a variety of programming challenges.
No comments yet.