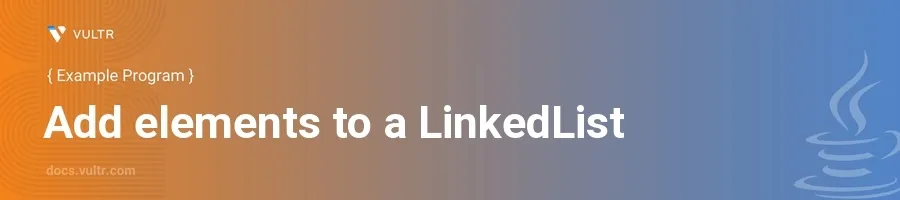
Introduction
The LinkedList
class in Java, part of the Java Collections Framework, provides a doubly linked list implementation of the List
and Deque
interfaces. This makes it an excellent choice for applications requiring frequent additions and deletions of list items. LinkedList
performs faster than ArrayList
when handling data dynamically due to its inherent structure, which allows elements to be added or removed without shifting the remainder of the data.
In this article, you will learn how to add elements to a LinkedList
in Java through practical examples. Discover methods to insert data at specific positions, append elements to the end of the list, and even add all elements from another collection.
Basics of Adding Elements to LinkedList
Add Elements at the End
Adding elements to the end of a LinkedList
utilizes the add(E e)
method, which appends the specified element to the end of this list.
Instantiate a
LinkedList
.Use the
add(E e)
method to add elements.javaLinkedList<String> fruits = new LinkedList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Cherry");
This block of code initializes a
LinkedList
of strings and adds three fruits to it. Each call toadd
places the new element at the end of the list.
Insert Elements at a Specific Position
Insert elements at a specified index within the list using add(int index, E element)
. This allows precise control over the element order.
Start with an existing
LinkedList
.Use the
add(int index, E element)
to insert an item.javaLinkedList<String> fruits = new LinkedList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add(1, "Mango"); // Inserts Mango before Banana
Here,
Mango
is inserted into theLinkedList
, positioned betweenApple
andBanana
. Using the index parameter, you can specify exactly where in the list the element should appear.
Advanced Techniques
Add Elements from Another Collection
To add all elements from another collection, such as an ArrayList
, to a LinkedList
, you use the addAll(Collection<? extends E> c)
method.
Create a
LinkedList
and a secondary collection.Use
addAll()
to import all elements from another collection.javaLinkedList<String> fruits = new LinkedList<>(); ArrayList<String> moreFruits = new ArrayList<>(); moreFruits.add("Kiwi"); moreFruits.add("Orange"); fruits.addAll(moreFruits);
In the example,
addAll()
method is used to add all elements frommoreFruits
ArrayList
into thefruits
LinkedList
. This is effective for merging lists.
Add Elements to the Beginning
Adding an element at the beginning of the list demonstrates the advantage of LinkedList
over ArrayList
in terms of performance.
Initialize a
LinkedList
.Use
addFirst(E e)
to add an element at the start of the list.javaLinkedList<String> fruits = new LinkedList<>(); fruits.add("Banana"); fruits.addFirst("Apple"); // Apple is now the first item
This snipped places
Apple
as the first item of the list, pushingBanana
to the second position.
Conclusion
Adding elements to a LinkedList
in Java is a flexible process, adaptable to various use cases from appending elements to integrating entire collections. The class provides multiple methods tailored for different insertion strategies, which you can choose based on the specific needs of your program. Utilize these examples to effectively manage lists in your Java applications, benefiting from the quick insertion and deletion capabilities of the LinkedList
data structure. As demonstrated, whether you are appending items to the end, inserting at a specific position, or merging from other collections, LinkedList
handles it efficiently.
No comments yet.