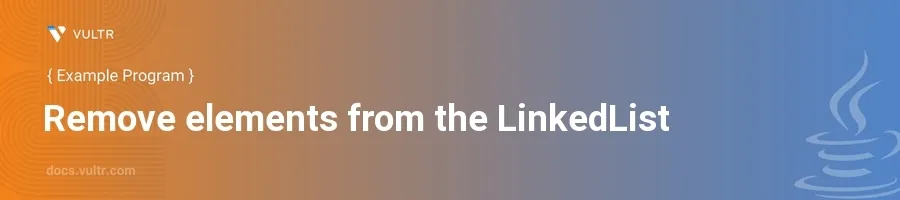
Introduction
The LinkedList
in Java represents a doubly-linked list data structure that enables efficient manipulation of elements at both the beginning and end of the list. It provides intuitive methods for dynamically modifying the collection, making it ideal for applications where frequent insertions and deletions occur.
In this article, you will learn how to remove elements from a LinkedList
in Java using various examples. Understand the different built-in methods available in the LinkedList
class, such as remove()
, removeFirst()
, removeLast()
, and how they can be utilized to modify list contents efficiently.
Removing Elements by Index
Remove Element at a Specific Index
Instantiate a
LinkedList
and add elements to it.Use the
remove(int index)
method to remove an element at a specific index.javaLinkedList<String> fruits = new LinkedList<>(); fruits.add("Apple"); fruits.add("Banana"); fruits.add("Cherry"); fruits.remove(1); // Removes "Banana" System.out.println(fruits);
This code first adds three elements to the
LinkedList
, then removes the element at index 1 ("Banana"). The resulting list contains "Apple" and "Cherry".
Exception Handling When Index is Out of Bounds
Always ensure the index provided to
remove(int index)
does not exceed the list's size.Handle possible
IndexOutOfBoundsException
.javatry { fruits.remove(5); // Attempt to remove an element at an out-of-bounds index } catch (IndexOutOfBoundsException e) { System.out.println("Error: " + e.getMessage()); }
In this code snippet, attempting to remove an element at index 5 throws an
IndexOutOfBoundsException
because it exceeds the size of the list. The error is caught and a message is printed.
Removing Elements by Value
Remove First Occurrence of Specific Element
Prepare a
LinkedList
with multiple identical items.Use
remove(Object o)
to remove the first occurrence of the specified element.javaLinkedList<String> animals = new LinkedList<>(); animals.add("Dog"); animals.add("Cat"); animals.add("Bird"); animals.add("Cat"); animals.remove("Cat"); // Removes the first occurrence of "Cat" System.out.println(animals);
Here, the
remove(Object o)
method removes the first "Cat" from the list. The remaining elements are "Dog", "Bird", and the second "Cat".
Using RemoveFirst() and RemoveLast()
Simplify Removing Elements at the Endpoints
Initialize a
LinkedList
and populate it with elements.Explore the
removeFirst()
andremoveLast()
methods to manage endpoint elements.javaLinkedList<Integer> numbers = new LinkedList<>(); numbers.add(10); numbers.add(20); numbers.add(30); numbers.removeFirst(); // Removes 10 numbers.removeLast(); // Removes 30 System.out.println(numbers);
The
removeFirst()
method eliminates the first element (10), andremoveLast()
removes the last element (30) from the list, leaving only 20.
Conclusion
The LinkedList
class in Java offers versatile methods for element deletion, catering to various needs like removing by index, value, or directly manipulating the first and last entries. Understanding and employing these methods elevates your ability to work efficiently with dynamic data in Java. Each method discussed, coupled with proper error handling, enhances control over list manipulation and ensures robust applications, especially in scenarios where rapid changes to data are frequent. Embrace these techniques to maintain concise, readable, and flexible code in your Java applications.
No comments yet.