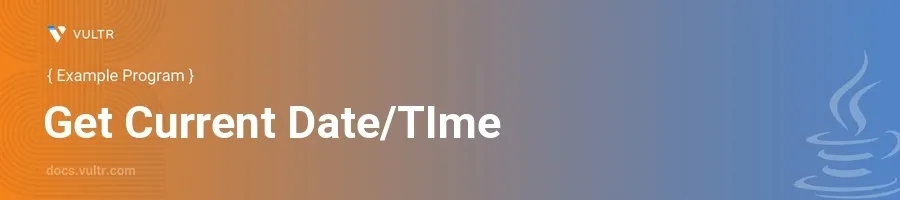
Introduction
When working with Java, you might often find yourself needing to handle dates and times, whether it's for logging, time-stamping, scheduling events, or other purposes. Java provides several ways to obtain the current date and time, each suited to different needs and complexities of the applications.
In this article, you will learn how to retrieve the current date and time in Java through various examples. Explore different classes like LocalDateTime
, ZonedDateTime
, Instant
, and Date
, and understand when and how to use each in your Java applications.
Using LocalDateTime
Retrieve Current Date and Time
Import the necessary package.
Use the
LocalDateTime.now()
methodjavaimport java.time.LocalDateTime; LocalDateTime currentDateTime = LocalDateTime.now(); System.out.println("Current Date and Time: " + currentDateTime);
This code snippet retrieves the current date and time using the
LocalDateTime
class. The output includes both the date and time without time zone information, making it ideal for applications not sensitive to time zone discrepancies.
Using ZonedDateTime
Get Current Date and Time with Time Zone
Import the
ZonedDateTime
class.Retrieve the current date and time in a specific time zone using
ZonedDateTime.now()
.javaimport java.time.ZonedDateTime; import java.time.ZoneId; ZonedDateTime zonedDatetime = ZonedDateTime.now(ZoneId.of("America/New_York")); System.out.println("Current Date and Time in New York: " + zonedDatetime);
This example shows how to get the current date and time considering the time zone. It's useful for applications that operate across multiple time zones and require precise time tracking.
Using Instant
Obtain Current GMT/UTC Date and Time
Import the
Instant
class.Use
Instant.now()
to get the GMT/UTC time.javaimport java.time.Instant; Instant timestamp = Instant.now(); System.out.println("Current GMT/UTC Time: " + timestamp);
Instant
represents a moment on the timeline in GMT/UTC. This is particularly useful for recording timestamps in an application that needs to align with international standards or requires coordination across different geological locations.
Using Date
Get Legacy Date and Time
Import the
Date
class fromjava.util
.Create an instance with
new Date()
, which captures the current date and time.javaimport java.util.Date; Date date = new Date(); System.out.println("Current Date and Time: " + date);
This snippet uses the older
Date
class fromjava.util
. It is simpler and direct but lacks the flexibility and timezone support provided by newer Java 8+ date-time classes.
Conclusion
Collecting the current date and time in Java can be managed through various methods, each suitable for specific requirements. Classes like LocalDateTime
, ZonedDateTime
, and Instant
provide robust support for handling time alongside their particular use cases in modern applications. Meanwhile, the Date
class offers a straightforward but less versatile approach, still used for backward compatibility and simpler needs. By implementing the techniques discussed, you can effectively manage date and time data to fulfill your application's needs while ensuring accuracy and efficiency.
No comments yet.