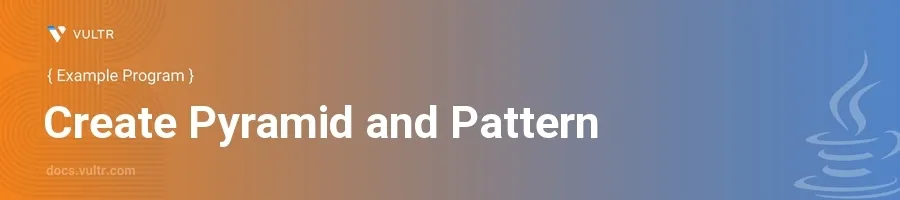
Introduction
Creating patterns and pyramids through programming not only enhances logical thinking but also helps in understanding nested loops and control structures. These types of exercises are common for beginners to improve their grasp of programming concepts such as iteration and conditional statements. Java, being a robust, object-oriented language, offers a structured way to create complex designs purely through code.
In this article, you will learn how to craft both simple and complex pyramid patterns using Java. Explore various methods to manipulate loops and conditional statements to generate aesthetically pleasing and symmetrical designs on your console. This knowledge will also assist in enhancing your problem-solving and algorithm development skills in Java.
Simple Pyramid Patterns
Creating a simple pyramid involves using nested loops where the outer loop handles the number of rows, and the inner loops manage the printing of spaces and stars or any other characters to shape the pyramid.
Creating a Simple Star Pyramid
Understand the logic required:
- Use an outer loop for each row of the pyramid.
- Within each row, implement two inner loops: one for spaces and one for stars.
Implement the Java code:
javapublic class StarPyramid { public static void main(String[] args) { int rows = 5; // Number of rows for the pyramid for (int i = 0; i < rows; i++) { for (int j = rows - i; j > 1; j--) { System.out.print(" "); // Printing space } for (int j = 0; j <= i; j++) { System.out.print("* "); // Printing star } System.out.println(); // Moving to the next line } } }
This code utilizes two loops inside a main loop that controls the row count. The first inner loop prints spaces needed to center the stars, and the second loop prints the stars.
Using Numeric Patterns for Pyramids
Adjust the previous logic to include numbers instead of stars:
- Replace the star with a number that increases with each column.
Code it in Java:
javapublic class NumberPyramid { public static void main(String[] args) { int rows = 5; for (int i = 1; i <= rows; i++) { for (int j = rows - i; j >= 1; j--) { System.out.print(" "); // Printing space } for (int j = 1; j <= i; j++) { System.out.print(i + " "); // Printing the current row number } System.out.println(); } } }
In this snippet, each row gets filled with the row's number rather than stars, creating a numeric pyramid.
Complex Pyramid Patterns
Creating more complex pyramid patterns often involves additional logic within the loops or even more complex loop structures.
Creating a Diamond Shape
Conceptualize the structure:
- A diamond shape can be thought of as two pyramids, one standing upright and the other upside down.
Write the Java program:
javapublic class DiamondPattern { public static void main(String[] args) { int rows = 5; // Total rows in the upper half of the diamond // Upper half of the diamond for (int i = 1; i <= rows; i++) { for (int j = rows - i; j >= 1; j--) { System.out.print(" "); // Print space } for (int j = 1; j <= 2 * i - 1; j++) { System.out.print("*"); // Print star } System.out.println(); } // Lower half of the diamond for (int i = rows - 1; i > 0; i--) { for (int j = 1; j <= rows - i; j++) { System.out.print(" "); // Print space } for (int j = 1; j <= 2 * i - 1; j++) { System.out.print("*"); // Print star } System.out.println(); } } }
This program first creates the upper half of the diamond using increasing numbers of stars, then the lower half by decreasing the count, thus forming a symmetrical diamond.
Conclusion
Creating pyramid and pattern programs in Java not only builds your understanding of loops and conditionals but also improves your overall programming logic and problem-solving skills. Start with simpler patterns and gradually move to complex designs. Each pattern offers unique challenges and learning opportunities. Keep experimenting with different shapes and designs to further refine your abilities in Java programming. By mastering these foundational programming patterns, you set a strong groundwork for more advanced programming projects and real-world applications.
No comments yet.