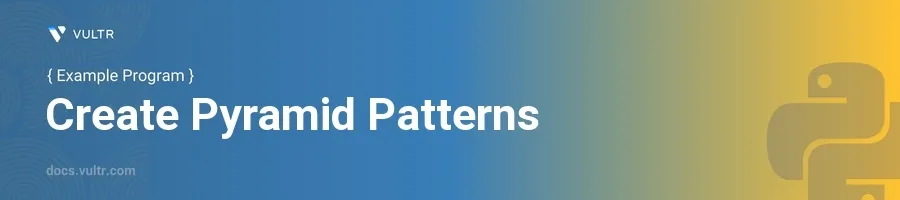
Introduction
Creating pyramid patterns in programming is a classic exercise that helps new developers understand nested loops and conditional statements better. It's a popular task in introductory programming courses and coding interviews, emphasizing control structure comprehension and basic syntax in Python.
In this article, you will learn how to create various pyramid patterns using Python. These examples will guide you through writing simple to more complex pyramid-shaped arrangements of asterisks, demonstrating each step with clear descriptions and code snippets.
Simple Pyramid Pattern
Create a Basic Pyramid
Initialize the number of pyramid levels.
Iterate through each level to print the appropriate number of asterisks.
pythondef pyramid(levels): for i in range(1, levels + 1): print(' ' * (levels - i) + '*' * (2 * i - 1)) pyramid(5)
This function,
pyramid
, creates a simple pyramid where each level has an increasing number of asterisks centered by spaces. For 5 levels, it produces a neatly centered pyramid.
Inverted Pyramid Pattern
Generate an Inverted Pyramid
Set the total number of levels in the inverted pyramid.
Use a loop to print descending numbers of asterisks.
pythondef inverted_pyramid(levels): for i in range(levels, 0, -1): print(' ' * (levels - i) + '*' * (2 * i - 1)) inverted_pyramid(5)
The
inverted_pyramid
function creates a pyramid that starts with the most considerable number of asterisks and decreases until it reaches one. Spacing is increased per line to keep the pattern centered.
Full Pyramid
Construct a Full Pyramid with Double Sides
Determine the height of the pyramid.
Use nested loops to print spaces and then stars, incrementing appropriately.
pythondef full_pyramid(levels): for i in range(1, levels + 1): # Print leading spaces print(' ' * (levels - i) + '*' * (2 * i - 1)) full_pyramid(5)
The
full_pyramid
functions similarly to the basic pyramid but can be adapted by adding additional characters or modifying patterns to create complex designs.
Diamond Shaped Pyramid
Create a Diamond Shape Using Asterisks
Define the total levels for the upper and lower patterns.
Construct the upper pyramid and then an inverted pyramid below it.
pythondef diamond_pyramid(levels): for i in range(1, levels + 1): print(' ' * (levels - i) + '*' * (2 * i - 1)) for i in range(levels - 1, 0, -1): print(' ' * (levels - i) + '*' * (2 * i - 1)) diamond_pyramid(5)
This function
diamond_pyramid
first prints a full pyramid followed by an inverted pyramid without the base row, creating a diamond shape in the console.
Conclusion
Pattern creation in Python leverages the fundamental concepts of loops and conditionals, allowing beginners and experienced programmers alike to practice core programming skills. From the simple to the more elaborate patterns like diamonds, each example provided adds complexity and challenges your understanding of Python syntax and logic. Use these patterns to enhance your problem-solving skills or as a creative element in your programming projects.
No comments yet.