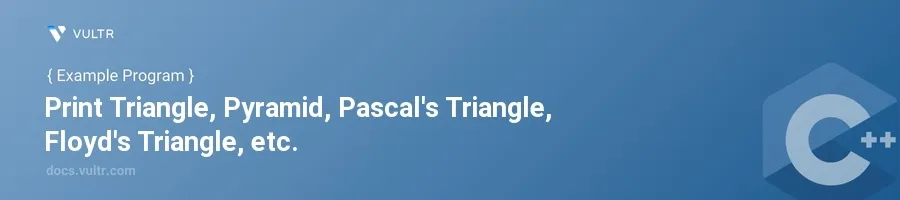
Introduction
C++ offers a versatile platform for creating a variety of patterns such as triangles, pyramids, Pascal's Triangle, and Floyd's Triangle. These geometric figures are not just visually appealing, but they also help in understanding the fundamentals of loops and conditionals in programming. Crafting these patterns develops logic and enhances problem-solving skills.
In this article, you will learn how to write C++ programs to draw different types of patterns—each type serving as an excellent exercise for understanding nested loops and algorithmic design. Explore the step-by-step approach to creating basic to complex patterns, improving your coding techniques along the way.
Creating a Simple Triangle
Making a simple right-angled triangle is a foundational skill in C++ pattern programming. The process involves using nested loops: the outer loop manages the rows, and the inner loop manages the columns or the number of characters per line.
Open your C++ development environment.
Include necessary headers and use the namespace.
Initialize the main function.
Declare and initialize variables for loop control and pattern size.
Construct nested loops to manage rows and characters per row.
cpp#include <iostream> using namespace std; int main() { int rows = 5; for (int i = 1; i <= rows; ++i) { for (int j = 1; j <= i; ++j) { cout << "* "; } cout << "\n"; } return 0; }
The outer loop iterates over the number of rows. The inner loop prints asterisks incrementally, increasing by one in each iteration to form a triangle shape.
Crafting a Pyramid Pattern
To create a centered pyramid, you adjust not only the number of characters per row but also manage spaces to center align the stars.
Write a program similar to the triangle but insert spaces before printing stars.
Adjust the inner loop to handle spaces decreasing as the number of stars increases.
cpp#include <iostream> using namespace std; int main() { int rows = 5; for (int i = 1; i <= rows; i++) { for (int j = 1; j <= rows - i; j++) { cout << " "; } for (int k = 1; k <= 2 * i - 1; k++) { cout << "* "; } cout << endl; } return 0; }
This code prints decreasing space characters and a centered pyramid of a specified number of rows.
Programming Pascal’s Triangle
Pascal's Triangle is a triangular array of the binomial coefficients. This pattern involves combinatorial logic but can still be rendered using loops.
Define function for factorial calculation.
Use the factorial to compute the combination formula for each element of the triangle.
Print each number based on its calculated combination values.
cpp#include <iostream> using namespace std; int factorial(int n) { int fact = 1; for (int i = 1; i <= n; i++) { fact *= i; } return fact; } int main() { int rows = 5; for (int i = 0; i < rows; i++) { for (int k = 0; k <= rows - i; k++) { cout << " "; } for (int j = 0; j <= i; j++) { cout << factorial(i) / (factorial(j) * factorial(i - j)) << " "; } cout << endl; } return 0; }
This snippet calculates the binomial coefficients for each position in Pascal's Triangle and prints them in a formatted manner.
Drawing Floyd’s Triangle
Floyd's Triangle is a right-angled triangular array of natural numbers, often introduced as an exercise in programming.
Implement a loop for rows.
Use a second loop to fill in increasing natural numbers.
Initialize a counter to keep track of the numbers.
cpp#include <iostream> using namespace std; int main() { int rows = 5, number = 1; for (int i = 1; i <= rows; i++) { for (int j = 1; j <= i; ++j) { cout << number << " "; ++number; } cout << endl; } return 0; }
Each new row starts where the previous row left off, creating a sequence of numbers formatted as a triangle.
Conclusion
Developing these patterns in C++ not only enhances your understanding of loops and conditions but also sharpens your algorithmic thinking. Start with simpler patterns like the right-angled triangle and gradually work your way up to more complex patterns like Pascal's and Floyd's Triangles. Experiment with variations of these basic patterns to further expand your proficiency in C++.
No comments yet.