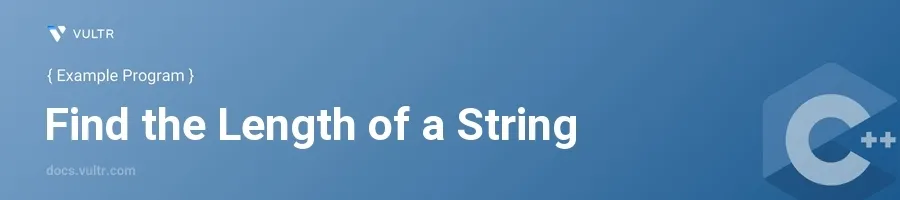
Introduction
Finding the string length is one of the fundamental operations of managing and manipulating strings in C++. It is used for various text processing operations, such as data validation, string manipulation, memory management, etc. C++ offers straightforward ways to find the length of a string in your programs.
In this article, you will learn different techniques to find the length of a string in C++. Discover how to use both the standard C++ library string class and the C-style string functions. Explore approaches that cover built-in functions and manual methods, ensuring you understand the internal workings and how you might implement these techniques in various applications.
Find String Length Using Standard C++ String Class
Find String Length Using string::length()
This method utilizes the C++ Standard Library's string class and its length()
method to determine the size of a string.
Include the string header.
Create an instance of string.
Use the
length()
method to find and print the string length.cpp#include <iostream> #include <string> int main() { std::string myString = "Hello, World!"; std::cout << "Length of the string is: " << myString.length() << std::endl; return 0; }
This code snippet introduces a string "Hello, World!" and then calculates its length using
myString.length()
. The result,13
, is then printed on the console.
Get String Size Using string::size()
This is another method offered by C++ string class that serves the same purpose as length()
but may be clearer in some contexts, particularly when dealing with collections.
Follow the same initial steps for including the string header and creating a string instance.
Use the
size()
method instead oflength()
to achieve the same result.cpp#include <iostream> #include <string> int main() { std::string myString = "Example text"; std::cout << "Length of the string is: " << myString.size() << std::endl; return 0; }
In this example, the
size()
method is used to determine the length of "Example text", which is12
.
Find String Length Using C-Style Strings
Find String Length with strlen()
strlen()
is a function from the C standard library <cstring>
, which calculates the length of a C-style string (null-terminated character array).
Include the cstring header.
Initialize a char array with a string.
Use
strlen()
to find the length.cpp#include <iostream> #include <cstring> int main() { char myString[] = "Another example"; std::cout << "Length of the string is: " << strlen(myString) << std::endl; return 0; }
This code evaluates the length of a C-style string "Another example", returning
15
as its length. Note thatstrlen()
calculates length by counting characters until it hits the null-terminator ('\0'
).
Manually Determining String Length in C++
Iterate Through Char Array
If you want a deeper understanding or need a custom implementation, calculating the length manually using a loop is an educational approach.
Create a character array initialized with your string.
Initialize a length counter to zero.
Iterate through the array, incrementing the counter until the null-terminator is reached.
cpp#include <iostream> int main() { char myString[] = "Manual Count"; int length = 0; while (myString[length] != '\0') { length++; } std::cout << "Length of the string is: " << length << std::endl; return 0; }
Here, each character of the array is checked in a while loop until the null-terminator. The length counter is incremented for each iteration, effectively calculating the string's length.
Conclusion
Exploring different methods to find the length of a string in C++ illustrates the versatility and power of the language. Whether using the high-level operations provided by the string class or diving into lower-level manipulation with C-style strings, C++ handles string operations effectively. By understanding these techniques, enhance the functionality and efficiency of your text processing applications.
No comments yet.