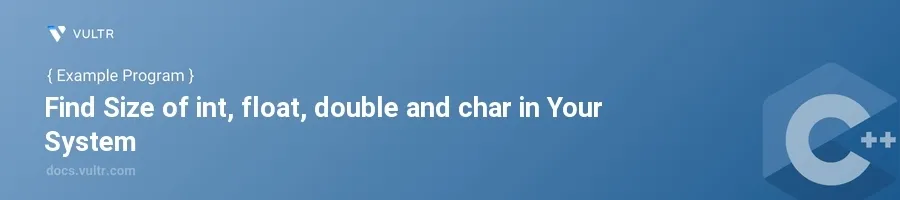
Introduction
In C++, different data types consume varying amounts of memory depending on the system and compiler used. This can affect not only the performance of an application but also its compatibility and portability across different hardware architectures. Understanding the size of basic data types like int, float, double, and char is crucial for optimizing memory usage and effectively managing data storage in C++ programs.
In this article, you will learn how to determine the size of fundamental data types in C++ by employing practical code examples. Grasp how to use these techniques to verify the data type sizes directly within your system.
Determining Size of Basic Data Types
Find Size of int
Initialize an integer variable.
Use the
sizeof
operator to determine its size.cpp#include<iostream> using namespace std; int main() { int intVariable; cout << "Size of int: " << sizeof(intVariable) << " bytes" << endl; return 0; }
This code snippet will output the size of an integer on your system. Note how
sizeof
directly queries the size ofintVariable
.
Find Size of float
Declare a float variable.
Apply the
sizeof
operator to find its size.cpp#include<iostream> using namespace std; int main() { float floatVariable; cout << "Size of float: " << sizeof(floatVariable) << " bytes" << endl; return 0; }
This example measures the memory used by a floating-point variable. The size is displayed in bytes.
Find Size of double
Create a double variable.
Measure its size using
sizeof
.cpp#include<iostream> using namespace std; int main() { double doubleVariable; cout << "Size of double: " << sizeof(doubleVariable) << " bytes" << endl; return 0; }
Here,
sizeof(doubleVariable)
helps determine the size occupied by a double data type in bytes.
Find Size of char
Declare a char variable.
Use the
sizeof
operator to check its size.cpp#include<iostream> using namespace std; int main() { char charVariable; cout << "Size of char: " << sizeof(charVariable) << " bytes" << endl; return 0; }
This code outputs the amount of memory a single character occupies, which is typically 1 byte across most systems and compilers, but verifying is always a good practice.
Conclusion
Understanding data sizes in C++ is essential for effectively managing memory and ensuring the optimal performance of your software. By utilizing the sizeof
operator, as demonstrated, you can easily check the size of various data types like int, float, double, and char directly on your system. Use these methods to adapt your application to various environments or to make informed choices when it comes to data type selection and memory usage. This approach ensures your C++ programs are both efficient and portable across different platforms.
No comments yet.