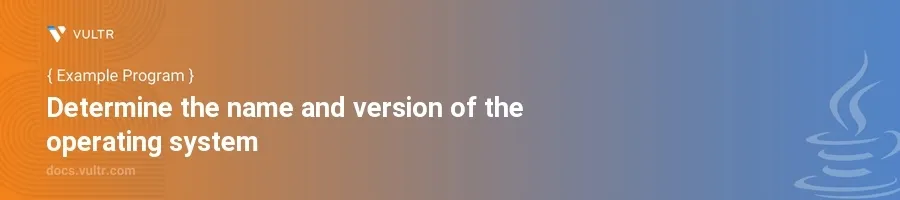
Introduction
Java provides a straightforward approach to accessing system properties, allowing programs to determine various runtime environment details such as the operating system name and version. This capability is especially useful for applications that need to adjust their behavior based on the OS they are running on or for gathering system diagnostics.
In this article, you will learn how to use Java to retrieve and display the name and version of the operating system. Examples are provided to help you understand how to implement these solutions in any Java program efficiently.
Retrieving OS Properties in Java
Accessing System Information
- Understand the importance of the
System
class. - Recognize that the
System.getProperty()
method is key to retrieving operating system information.
Display OS Name and Version
Set up a Java environment to write your code.
Use the following example to retrieve and display the operating system name and version:
javapublic class OSInfo { public static void main(String[] args) { String osName = System.getProperty("os.name"); String osVersion = System.getProperty("os.version"); System.out.println("Operating System Name: " + osName); System.out.println("Operating System Version: " + osVersion); } }
This code initializes two string variables,
osName
andosVersion
, by fetching the respective system properties. The properties"os.name"
and"os.version"
are predefined system property keys that Java recognizes and uses to return the appropriate system information. The resulting strings are then printed to the console.
Handling Potential Errors
- Consider the possibility of a security exception.
- Prepare your code to handle scenarios where security settings prevent access to system properties.
Advanced Usage
Developing Conditionally Based on OS
Further emphasize the practical application of OS information.
Modify the existing code to behave differently across different operating systems:
javapublic class OSCondition { public static void main(String[] args) { String osName = System.getProperty("os.name").toLowerCase(); if(osName.contains("windows")) { System.out.println("This is a Windows operating system."); } else if(osName.contains("mac")) { System.out.println("This is a Mac operating system."); } else if(osName.contains("linux")) { System.out.println("This is a Linux operating system."); } else { System.out.println("Operating system not recognized!"); } } }
This example extends the initial program by incorporating conditional logic based on the operating system's name. It converts the name of the OS to lower case to ensure case-insensitive comparison, which enhances the robustness of the code.
Using Results in Application Logic
- Illustrate practical integration of OS check results into an application.
- Brainstorm scenarios where you might need different actions or settings depending on the operating system, such as file paths, operating procedures, or compatibility modes.
Conclusion
Retrieving and using the operating system's name and version in Java is both straightforward and immensely useful. Whether you need this information for conditional behavior, system diagnostics, logging, or user interface adjustments, Java's built-in system properties provide a reliable method. By mastering the use of System.getProperty()
for accessing OS information and integrating it according to your application's needs, you ensure that your software can adapt to different environments effectively.
No comments yet.